React Fragment and How To Use It?
Rendering multiple sibling components dynamically is a widespread practice in React. To preserve the tree structure of HTML elements when returning JSX from a React component, we must ensure that the component returns a maximum of one top-level node. Fragments provide a highly effective solution to this issue.
Let's see what we can do with fragments.
Let’s start by understanding the problem that Fragments are meant to solve.
function App() {
return (
<div>
<ul>
<Students />
</ul>
</div>
);
}
export default App;
const Students = () => {
return (
<li>Foo</li>
<li>Bar</li>
<li>John</li>
)
}
This piece of code has an error that lies in the Students component.
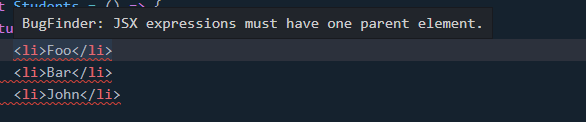
Here our IDE is indicating an error that 'JSX expressions must have one parent element'. We attempted to return three elements with no parent, which resulted in the code failing to compile. To resolve this issue, we need to enclose these elements within a container. For the time being we are using div-
function App() {
return (
<div>
<ul>
<Students />
</ul>
</div>
);
}
export default App;
const Students = () => {
return (
<div>
<li>Foo</li>
<li>Bar</li>
<li>John</li>
</div>
)
}
Now the error gone and everything seemingly fine, let's take a look at the DOM structure of our application. Do you observe anything unusual?
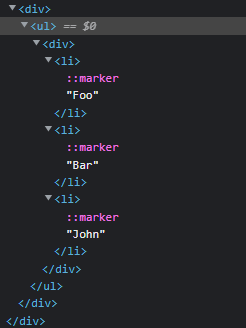
You're right, the "li" elements are wrapped inside an extra "div" element that we had to add. Instead of using "div," we can use "Fragment."
Now let's try it out using React fragment:
import React from "react";
function App() {
return (
<div>
<ul>
<Students />
</ul>
</div>
);
}
export default App;
const Students = () => {
return (
<React.Fragment>
<li>Foo</li>
<li>Bar</li>
<li>John</li>
</React.Fragment>
)
}
Let’s check out the DOM structure again to see if the problem went away.
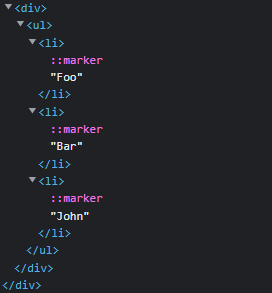
Can you distinguish the difference? Fragments enable us to group nodes without generating an extra element in the DOM, making it a highly useful tool.
For most use cases, we can utilize a concise syntax. We can employ it in the same manner as any other element.
Instead of using <React.Fragment>…</React.Fragment>, we can use the shorthand <>…</>.
import React from "react";
function App() {
return (
<div>
<ul>
<Students />
</ul>
</div>
);
}
export default App;
const Students = () => {
return (
<>
<li>Foo</li>
<li>Bar</li>
<li>John</li>
</>
)
}
I hope you've enjoyed this tutorial. Thanks for reading.