Generate Barcode in Laravel
A barcode or bar code is a method of representing data in a visual, machine-readable form. In this article, we’re going to generate barcode in Laravel. I’m testing on Laravel 8.9.0. Let’s get started:
Table of Contents
- Install Laravel and Basic Configurations
- Install Package & Configure
- Create Controller
- Create View File
- Define Route
- Run & Test
Install Laravel and Basic Configurations
Each Laravel project needs this thing. That’s why I have written an article on this topic. Please see this part from here: Install Laravel and Basic Configurations.
Install Package & Configure
Install milon/barcode package in the project:
composer require milon/barcode
Laravel 5.5 uses package auto-discovery, so doesn’t require you to manually add the service provider & aliases. If you don’t use auto-discovery, add the service provider & aliases in config/app.php
file like:
'providers' => [
....
Milon\Barcode\BarcodeServiceProvider::class,
],
'aliases' => [
....
'DNS1D' => Milon\Barcode\Facades\DNS1DFacade::class,
'DNS2D' => Milon\Barcode\Facades\DNS2DFacade::class,
]
Create Controller
Run this command to create a barcode controller:
php artisan make:controller BarCodeController
Now open the controller and paste this code:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class BarCodeController extends Controller
{
// index
public function index()
{
return view('barcode');
}
}
Create View File
Make a blade view file named barcode.blade.php and paste the below code:
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<title>Generate Barcode in Laravel - MyNotePaper.com</title>
</head>
<body>
<div class="container text-center" style="margin-top: 50px;">
<h3 class="mb-5">Generate Barcode in Laravel</h3>
<div>{!! DNS1D::getBarcodeHTML('4445645656', 'C39') !!}</div></br>
<div>{!! DNS1D::getBarcodeHTML('4445645656', 'POSTNET') !!}</div></br>
<div>{!! DNS1D::getBarcodeHTML('4445645656', 'PHARMA') !!}</div></br>
<div>{!! DNS2D::getBarcodeHTML('4445645656', 'QRCODE') !!}</div></br>
</div>
</body>
</html>
Define Route
Open routes/web.php and register this route:
use App\Http\Controllers\BarCodeController;
Route::get('/barcode', [BarcodeController::class, 'index']);
Run & Test
Now run the project, visit /barcode
route & test.
// run application
php artisan serve
// visit the route
http://localhost:8000/barcode
Output:
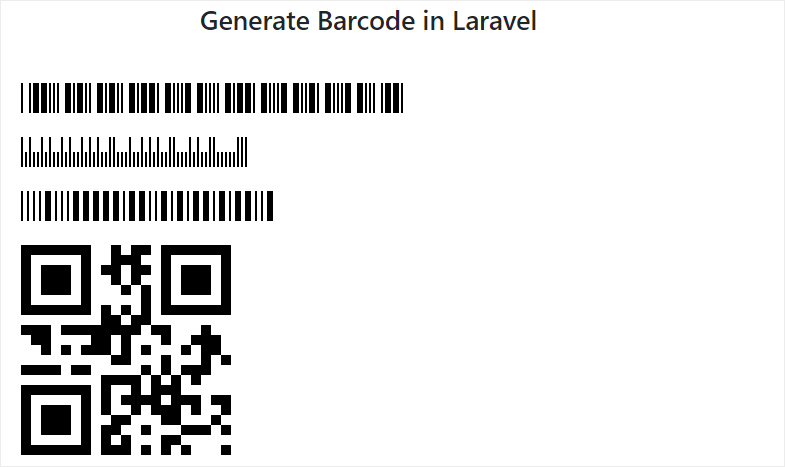
Note: We can set width and height, color, add text, 1 & 2D barcodes etc. using this package. Have a look at milon/barcode GitHub repository to see more examples.
That’s all, folks. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.