Chapter 18 - What is ResourceClass and How to Format API with ResourceClass
Hello Artisan's, welcome to the 11th chapter of being an Artisanary. In this chapter we'll learn what is ResourceClass and how can we format/organize our API in a convenient way. So if you already complete the previous chapters/sections, then you're good to go, if not my recommendation would be please complete the previous chapters. Because we'll use the same old repository that we use in chapter 4. Let's move on to our topic.
Note: Tested on Laravel 10.0
In Laravel, a resource class is a PHP class that provides a convenient way to organize and manage the logic related to a specific API resource. It can be used to define the response format for various HTTP requests made to the API endpoints.
Using resource classes in Laravel can help you to:
- centralize your code related to handling responses for API resources
- make your code more organized and easier to maintain
- simplify your API responses by defining a standard format for all endpoints
Format API with ResourceClass
At first we need to create a resource class by firing the below command in the terminal.
php artisan make:resource BlogResource
It'll create a file under app/Http/Resources/BlogResource.php, now we need to define the in toArray() method. Look at the below source code.
<?php
namespace App\Http\Resources;
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\JsonResource;
class BlogResource extends JsonResource
{
public function toArray(Request $request): array
{
return [
'id' => $this->id,
'title' => $this->title,
'description' => $this->description,
'image' => asset($this->image),
];
}
}
Now we need to call the above created and formatted BlogResource in our controller. So, let's modify the index() method of previously created Api/BlogController.
public function index(): \Illuminate\Http\JsonResponse
{
try {
$data = [
'blogs' => BlogResource::collection($this->blogRepository->all())
];
return response()->json($data);
} catch (\Exception $e) {
return response()->json([
'error' => $e->getMessage()
]);
}
}
It's time for see the output. Now if you hit the Send button you'll find the below output
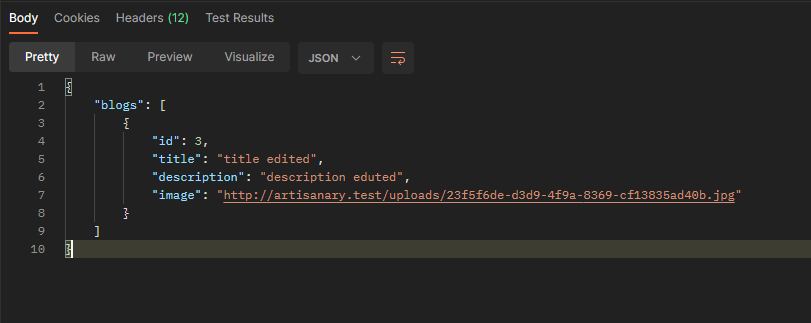
This way you can organize your API in any format you want. And yes most of the developers foreach the API in their controller. But this is not a good habit. If you format your API in controller every time when this API is required you need to format again. But using ResourceClass, you can use it anywhere you want.
So, that's an end of our long series here. And yes keep up to date with the Github repository and I also add this PostmanCollection is GitHub repo as well. So, that you can import and test as well. It's time to say goodbye. And yes if you've any feedback or comment, please don't hesitate to ask. See you again. Happy coding ๐.