Build and Run Vue.js Application with Docker
In this article, I’m going to dockerize Vue application. We know that Docker is a set of platform-as-a-service (PaaS) products that use OS-level virtualization to deliver software in packages called containers. Let’s build and run app with Docker.
You can take a look at basic docker commands.
Table of Contents
Step 1 : Create a Vue Project
You need to install Vue CLI if not installed on your PC.
npm install -g @vue/cli
Then run this command to create a Vue app:
# create project
vue create hellovue
# go to project folder
cd hellovue
Step 2 : Create Dockerfile
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. Create a file named Dockerfile at the root of your project. Now we’re are going to write some commands in the Dockerfile to run our project.
# develop stage
FROM node:alpine as develop-stage
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
# build stage
FROM develop-stage as build-stage
RUN npm run build
# production stage
FROM nginx:alpine as production-stage
COPY --from=build-stage /app/dist /usr/share/nginx/html
EXPOSE 90
CMD ["nginx", "-g", "daemon off;"]
FROM node:alpine
means, Docker will pull nodejs alpine version from Docker Hub. Alpine makes a great docker container, because it is so small and optimized to be run in RAM.
Step 3 : Make .dockerignore File
.dockerignore is to prevent files from being added to the initial build context that is sent to the Docker daemon when we do docker build. Here’s an example:
.git
.DS_Store
node_modules
dist
Step 4 : Build and Run Container
We’ll build the project as a Docker container with this command:
docker build -t hellovue .
Now let’s run the container at port 90:
docker run -p 90:80 hellovue
Visit http://localhost:90
and see the output like this:
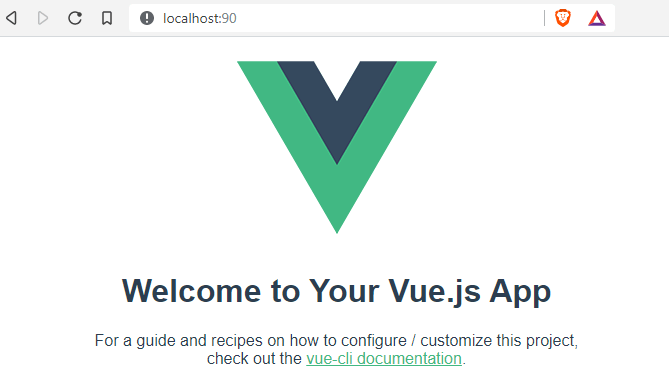
We can also run the container with some attributes. Have a look at this page.
The tutorial is over. You can download this project from GitHub. Thank you.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.