Laravel Strong Password Validation
In this article, we are going to learn how to set strong password validation rule in Laravel. Let’s get started:
Table of Contents
Make a Rule
We are going to create a new rule called IsValidPassword
. Run this comand:
php artisan make:rule IsValidPassword
Open the new rule IsValidPassword.php file from app/Rules folder and paste this code:
<?php
namespace App\Rules;
use Illuminate\Support\Str;
use Illuminate\Contracts\Validation\Rule;
class IsValidPassword implements Rule
{
/**
* Determine if the Length Validation Rule passes.
*
* @var boolean
*/
public $lengthPasses = true;
/**
* Determine if the Uppercase Validation Rule passes.
*
* @var boolean
*/
public $uppercasePasses = true;
/**
* Determine if the Numeric Validation Rule passes.
*
* @var boolean
*/
public $numericPasses = true;
/**
* Determine if the Special Character Validation Rule passes.
*
* @var boolean
*/
public $specialCharacterPasses = true;
/**
* Determine if the validation rule passes.
*
* @param string $attribute
* @param mixed $value
* @return bool
*/
public function passes($attribute, $value)
{
$this->lengthPasses = (Str::length($value) >= 8);
$this->uppercasePasses = (Str::lower($value) !== $value);
$this->numericPasses = ((bool)preg_match('/[0-9]/', $value));
$this->specialCharacterPasses = ((bool)preg_match('/[^A-Za-z0-9]/', $value));
return ($this->lengthPasses && $this->uppercasePasses && $this->numericPasses && $this->specialCharacterPasses);
}
/**
* Get the validation error message.
*
* @return string
*/
public function message()
{
switch (true) {
case !$this->uppercasePasses
&& $this->numericPasses
&& $this->specialCharacterPasses:
return 'The :attribute must be at least 8 characters and contain at least 1 uppercase character.';
case !$this->numericPasses
&& $this->uppercasePasses
&& $this->specialCharacterPasses:
return 'The :attribute must be at least 8 characters and contain at least 1 number.';
case !$this->specialCharacterPasses
&& $this->uppercasePasses
&& $this->numericPasses:
return 'The :attribute must be at least 8 characters and contain at least 1 special character.';
case !$this->uppercasePasses
&& !$this->numericPasses
&& $this->specialCharacterPasses:
return 'The :attribute must be at least 8 characters and contain at least 1 uppercase character and 1 number.';
case !$this->uppercasePasses
&& !$this->specialCharacterPasses
&& $this->numericPasses:
return 'The :attribute must be at least 8 characters and contain at least 1 uppercase character and 1 special character.';
case !$this->uppercasePasses
&& !$this->numericPasses
&& !$this->specialCharacterPasses:
return 'The :attribute must be at least 8 characters and contain at least 1 uppercase character, 1 number, and 1 special character.';
default:
return 'The :attribute must be at least 8 characters.';
}
}
}
Apply the Rule
Now we can apply the rule in any password field. Let’s apply in RegisterController. Open the controller from app/Http/Controller/Auth folder and apply the rule like:
use App\Rules\IsValidPassword;
protected function validator(array $data)
{
return Validator::make($data, [
'name' => ['required', 'string', 'max:255'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
'password' => ['required', 'string', 'confirmed', new isValidPassword()],
]);
}
Output
Run the application and test registration form. You’ll notice validation error like this:
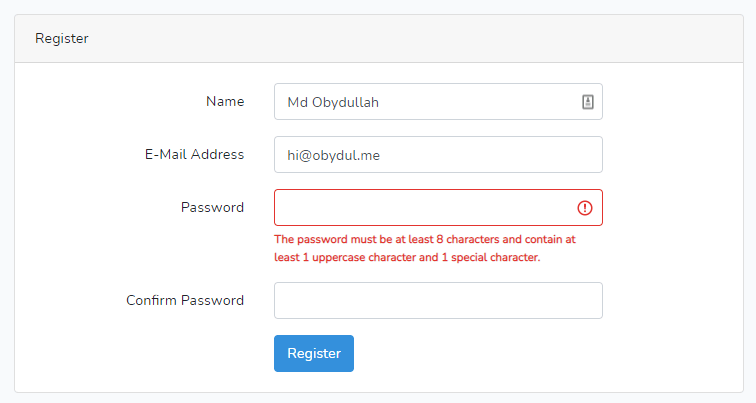
That’s all, folks. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.