Laravel Hide .env Variables and Details From Debug Output
In this tutorial, I’m going to share how to hide .env variables from Laravel error page. Let’s get started:
Table of Contents
Hide Specific Variables
From Laravel 5.5.13, we’re able to hide variables by by listing them under the key debug_blacklist
in config/app.php
. For example, given this config:
return [
// ...
'debug_blacklist' => [
'_ENV' => [
'APP_KEY',
'DB_PASSWORD',
'REDIS_PASSWORD',
'MAIL_PASSWORD',
'PUSHER_APP_KEY',
'PUSHER_APP_SECRET',
],
'_SERVER' => [
'APP_KEY',
'DB_PASSWORD',
'REDIS_PASSWORD',
'MAIL_PASSWORD',
'PUSHER_APP_KEY',
'PUSHER_APP_SECRET',
],
'_POST' => [
'password',
],
],
];
Results in this output:
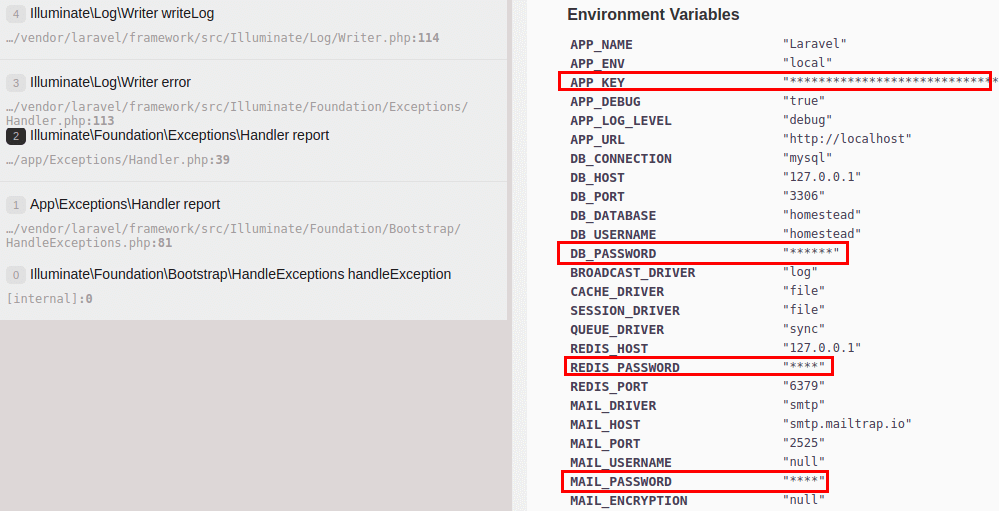
Hide All Variables
We’re able to hide all variables like this:
return [
// ...
'debug_blacklist' => [
'_COOKIE' => array_keys($_COOKIE),
'_SERVER' => array_keys($_SERVER),
'_ENV' => array_keys($_ENV),
],
];
Results in this output:
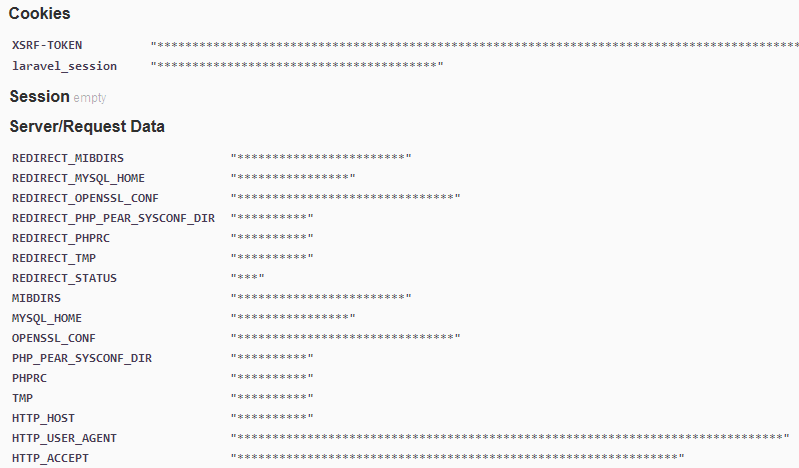
Hide Except Variables
If we need to whitelist a property or two, we ca do so:
return [
// ...
'debug_blacklist' => [
'_COOKIE' => array_diff(
array_keys($_COOKIE),
explode(",", env('DEBUG_COOKIE_WHITELIST', ""))
),
'_SERVER' => array_diff(
array_keys($_SERVER),
explode(",", env('DEBUG_SERVER_WHITELIST', ""))
),
'_ENV' => array_diff(
array_keys($_ENV),
explode(",", env('DEBUG_ENV_WHITELIST', ""))
),
],
];
Then in your .env, add this variables:
DEBUG_SERVER_WHITELIST="APP_URL,QUERY_STRING"
Stop Debugging
To make .env variables 100% secure, you should turn off debugging. In .env file, make:
APP_DEBUG=false
That’s it. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.