Generate JSON using SortableJS Drag and Drop
In this guide, I’m going to show you how to generate JSON using Sortable drag and drop. Let begin:
Table of Contents
- Introduce with SortableJS
- Make a Simple List
- Define Sortable Class
- Convert Products Array to JSON
- The Full Code
- The Output
Step 1 : Introduce with SortableJS
In short, SortableJS is a JavaScript library for reorderable drag-and-drop lists. It works in Meteor, AngularJS, React, Polymer, Vue, Ember, Knockout and any CSS library.
In this tutorial, I will use their CDN. Here’s the CDN URL:
<script src="https://raw.githack.com/SortableJS/Sortable/master/Sortable.js"></script>
Step 2 : Make a Simple List
Let’s make a simple list. I’m going to make a simple product list. Here’s the code:
<div id="productList" class="list-group list-group-flush">
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">1</span>. <span id="title[]">£50 PSN PlayStation Network Card UK</span></span>
<span class="badge badge-primary badge-pill">21</span>
</li>
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">2</span>. <span id="title[]">Microsoft Office 2019 Professional Plus</span></span>
<span class="badge badge-primary badge-pill">35</span>
</li>
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">3</span>. <span id="title[]">FIFA 20 Standard Edition Xbox One</span></span>
<span class="badge badge-primary badge-pill">45</span>
</li>
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">4</span>. <span id="title[]">Apex Legends 6700 Coins PS4</span></span>
<span class="badge badge-primary badge-pill">17</span>
</li>
</div>
Step 3 : Define Sortable Class
We have created a product list with ID productList. Let’s define the simple Sortable class:
<script>
// sortable
Sortable.create(productList, {
group: "sorting",
sort: true
});
</script>
Step 4 : Convert Products Array to JSON
Sortable doesn’t require jQuery. I’m going to include jQuery to convert Array to JSON. Here’s my jQuery code:
<script>
// generate product JSON
$('#generateJSON').click(function() {
let data = {};
var titles = $('span[id^=title]').map(function(idx, elem) {
return $(elem).text();
}).get();
data['products'] = titles;
// encode to JSON format
var products_json = JSON.stringify(data,null,'\t');
$('#printCode').html(products_json);
});
</script>
Step 5 : The Full Code
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Hello, world!</title>
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<!-- Latest Sortable -->
<script src="https://raw.githack.com/SortableJS/Sortable/master/Sortable.js"></script>
<!-- jQuery -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<style>
body {
padding: 20px;
}
.list-group-item {
cursor: move;
cursor: -webkit-grabbing;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-6">
<h4 class="text-center">Products</h4>
<!-- product list -->
<div id="productList" class="list-group list-group-flush">
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">1</span>. <span id="title[]">£50 PSN PlayStation Network Card UK</span></span>
<span class="badge badge-primary badge-pill">21</span>
</li>
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">2</span>. <span id="title[]">Microsoft Office 2019 Professional Plus</span></span>
<span class="badge badge-primary badge-pill">35</span>
</li>
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">3</span>. <span id="title[]">FIFA 20 Standard Edition Xbox One</span></span>
<span class="badge badge-primary badge-pill">45</span>
</li>
<li class="list-group-item d-flex justify-content-between align-items-center">
<span><span class="font-weight-bold">4</span>. <span id="title[]">Apex Legends 6700 Coins PS4</span></span>
<span class="badge badge-primary badge-pill">17</span>
</li>
</div>
<br>
<div class="text-center">
<button type="button" id="generateJSON" class="btn btn-info">Generate JSON</button>
</div>
</div>
<div class="col-md-6">
<h4 class="text-center">JSON</h4>
<pre id="printCode"></pre>
</div>
</div>
</div>
<script>
// generate product JSON
$('#generateJSON').click(function() {
let data = {};
var titles = $('span[id^=title]').map(function(idx, elem) {
return $(elem).text();
}).get();
data['products'] = titles;
// encode to JSON format
var products_json = JSON.stringify(data,null,'\t');
$('#printCode').html(products_json);
});
</script>
<script>
// sortable
Sortable.create(productList, {
group: "sorting",
sort: true
});
</script>
</body>
</html>
Step 6 : The Output
Let’s run this file and see the output:
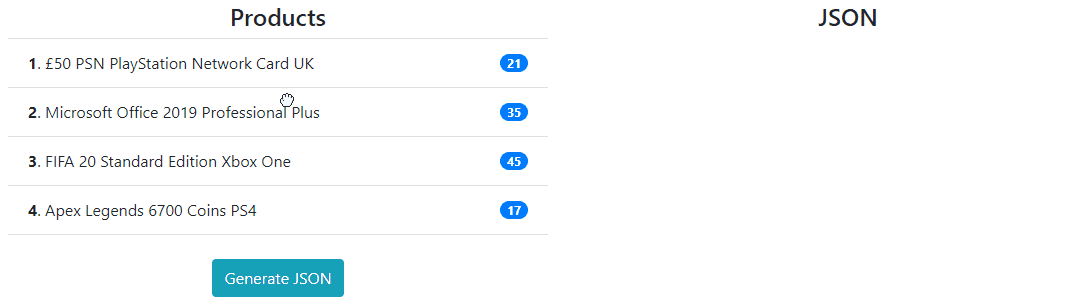
The topic is over. You will find more information from GitHub. Thank you
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.