JavaScript Validate User Inputs
Hello dev's, today I'll show you how to create a dropdown using JS & CSS only, without using any framework like bootstrap. So, let's see how we can easily create a dropdown using JS & CSS only.
Here we we'll write some CSS code and JS code to manipulate a html content. So, look at the below source code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Validating user Inputs using JavaScript - shouts.dev</title>
</head>
<body>
<form id="myForm">
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<button type="submit">Submit</button>
</form>
<div id="error" style="color : red"></div>
<script>
const form = document.getElementById('myForm');
const emailInput = document.getElementById('email');
const errorDiv = document.getElementById('error');
function validateEmail(email) {
const re = /^([a-zA-Z0-9._-]+)@([a-zA-Z0-9.-]+)\.([a-zA-Z]{2,})$/;
return re.test(email);
}
form.addEventListener('submit', function(event) {
event.preventDefault();
const email = emailInput.value;
if (validateEmail(email)) {
alert('Everything is fine, the form can be submitted');
form.submit();
} else {
errorDiv.textContent = 'Please enter a valid email address.';
}
});
</script>
</body>
</html>
Here, we created a simple form with a single input field for an email address. We have also added an error message element that will display if the user input is not valid.
We have written a validation function called validateEmail() that uses a regular expression to check if the email address input matches the expected format. We have then added an event listener to the form that prevents the default form submission behavior and instead calls the validateEmail() function on the email input value. If the input is valid, an alert will be given saying “Everything is fine, the form can be submitted” and the form will be submit. If the input is not valid, an error message is displayed in the errorDiv element.
So if we open our html file in our browser then we can find the below output.
With validation message
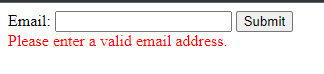
And when the form is submitted successfully
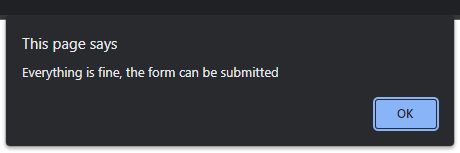
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. 🙂