Dockerize a Laravel App With Apache, MariaDB Within a Few Minutes
Hi artisans! In this article, I’m going to show how to dockerize a Laravel application with Apache, MariaDB in a few minutes. The docker and docker compose are must be installed on your machine. You can download those from their official website. Let’s get started:
Table of Contents
- Create Dockerfile
- Create Docker Compose File
- Update .env File
- Build and Run
- Run Artisan, NPM Commands in Container
- Check Database Connection
Create Dockerfile
Navigate to the root directory of your Laravel application. Then create a folder named .docker. Under the .docker folder, create two files named Dockerfile and vhost.conf.
Open Dockerfile file and paste this code:
FROM php:7.4.1-apache
USER root
WORKDIR /var/www/html
RUN apt update && apt install -y \
nodejs \
npm \
libpng-dev \
zlib1g-dev \
libxml2-dev \
libzip-dev \
libonig-dev \
zip \
curl \
unzip \
&& docker-php-ext-configure gd \
&& docker-php-ext-install -j$(nproc) gd \
&& docker-php-ext-install pdo_mysql \
&& docker-php-ext-install mysqli \
&& docker-php-ext-install zip \
&& docker-php-source delete
COPY .docker/vhost.conf /etc/apache2/sites-available/000-default.conf
RUN curl -sS https://getcomposer.org/installer | php -- --install-dir=/usr/local/bin --filename=composer
RUN chown -R www-data:www-data /var/www/html && a2enmod rewrite
Open vhost.conf file and paste this code:
<VirtualHost *:80>
DocumentRoot /var/www/html/public
<Directory "/var/www/html">
AllowOverride all
Require all granted
</Directory>
#ErrorLog ${APACHE_LOG_DIR}/error.log
#CustomLog ${APACHE_LOG_DIR}/access.log combined
</VirtualHost>
Create Docker Compose File
Go to root directory and create a file called docker-compose.yml. Open the newly created file and paste this config:
version: '3.7'
services:
app:
build:
context: .
dockerfile: .docker/Dockerfile
image: 'shouts.dev/laravel'
container_name: shouts-laravel-app
ports:
- "80:80"
volumes:
- ./:/var/www/html
networks:
- laravel-shouts
depends_on:
- mysql
mysql:
image: 'mariadb:latest'
container_name: shouts-laravel-db
restart: unless-stopped
ports:
- "3306:3306"
environment:
MYSQL_DATABASE: ${DB_DATABASE}
MYSQL_ROOT_PASSWORD: ${DB_PASSWORD}
MYSQL_PASSWORD: ${DB_PASSWORD}
MYSQL_USER: ${DB_USERNAME}
MYSQL_ALLOW_EMPTY_PASSWORD: 'yes'
volumes:
- ./database/dbdata:/var/lib/mysql
networks:
- laravel-shouts
networks:
laravel-shouts:
driver: bridge
Update .env File
In the .env file, we have to change this line DB_HOST=127.0.0.1
to DB_HOST=mysql
. Because in the docker-compose.yml file, we named our database service as mysql. So, it’ll look like:
DB_CONNECTION=mysql
DB_HOST=mysql
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=root
DB_PASSWORD=
[Optional]: Add this line /database/dbdata to .gitignore file.
Build and Run
The configuration is done. Our application is ready to run in the docker container. Run this command to build our app’s docker image and run it as a container:
docker-compose up -d
The first time, it may take a few minutes. Because docker will pull all dependencies in our system. After creating the image, run this command to see our app’s containers:
docker ps

Our application is running on docker. Visit localhost
or SERVER_IP
to see your application.
Run Artisan, NPM Commands in Container
We can run any commands in our docker container. Here’s the structure to run the command:
# structure
docker exec <container-name/ID> php artisan --version
# example
docker exec b2fe1b8dac0f php artisan --version
docker exec shouts-laravel-app php artisan --version
We can also login to container and then run commands:
# structure
docker exec -it <container-name/ID> bash
# then run any commands
php artisan --version
npm install && npm run dev
Check Database Connection
To check our app is connected to MariaDB/MySQL, run these commands:
# structure
docker exec -it <container-name/ID> bash
# then run
php artisan tinker
DB::connection()->getDatabaseName();
You’ll see the database name like:
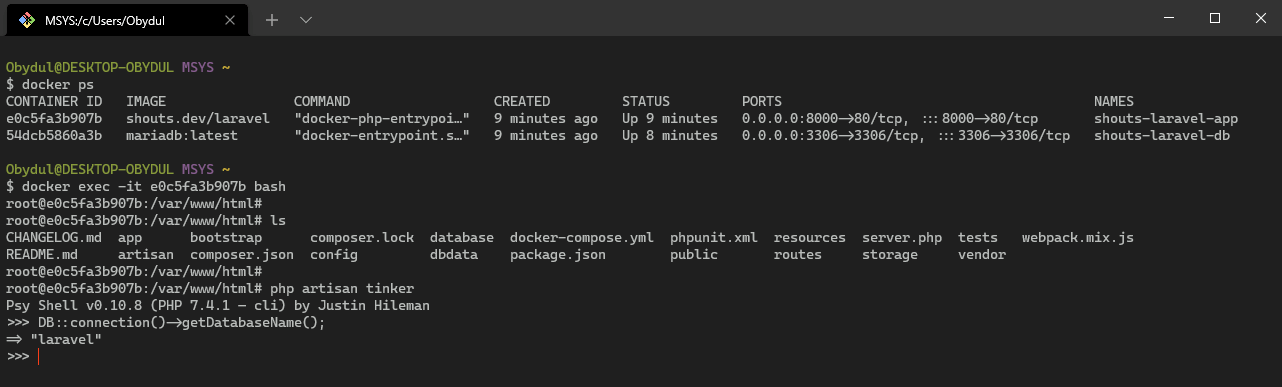
Note: You can take a look at “Basic Docker Commands at a Glance” article.
That’s all. You can download this project from GitHub. Thanks for reading. 🙂
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.