Laravel 10 Generate PDF and Send Email Example
Hello Artisan, today I'll show you How Generate PDF and Send Email Example in our Laravel application. Learn how to use dompdf to generate PDFs and send them as email attachments in Laravel 10. Discover how to send emails with PDF attachments in Laravel 10.Explore the process of generating PDFs in Laravel 10 and sending them via email.
- Install Laravel
- Install dompdf Package
- Make Configuration
- Create Mail Class
- Add Controller
- Add Route
- Create View File
- Run Laravel App
Creating the Laravel app is not necessary for this step, but if you haven't done it yet, you can proceed by executing the following command
composer create-project --prefer-dist laravel/laravel Demp-Mail
To install the barryvdh/laravel-dompdf package in your Laravel application, you can use Composer by running the following command:
Firstly, we'll install the 'barryvdh/laravel-dompdf' package in your Laravel application using Composer.
composer require barryvdh/laravel-dompdf
In the first step, you have to add send mail configuration with mail driver, mail host, mail port, mail username, and mail password so Laravel will use those sender details on the email. So you can simply add the following.
MAIL_MAILER=smtp
MAIL_HOST=sandbox.smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=93d8c1571f2ecc
MAIL_PASSWORD=95ae9fdfbc3235
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME="${APP_NAME}"
We are going from scratch and in first step, we will create email for testing using Laravel Mail facade. So let's simple run bellow command.
php artisan make:mail MailExample
You should find a new folder named "Mail" within the app directory. In this folder, there will be a file called “MailExample.php.” Copy the code provided below. Navigate to the "Mail" folder in the app directory. Open the "MailExample.php" file. Paste the previously copied code into the "MailExample.php" file.
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
use Illuminate\Mail\Mailables\Attachment;
class MailExample extends Mailable
{
use Queueable, SerializesModels;
public $mailData;
/**
* Create a new message instance.
*/
public function __construct($mailData)
{
$this->mailData = $mailData;
}
/**
* Get the message envelope.
*/
public function envelope(): Envelope
{
return new Envelope(
subject: $this->mailData['title'],
);
}
/**
* Get the message content definition.
*/
public function content(): Content
{
return new Content(
view: 'emails.myTestMail',
with: $this->mailData
);
}
/**
* Get the attachments for the message.
*
* @return array
*/
public function attachments(): array
{
return [
Attachment::fromData(fn () => $this->mailData['pdf']->output(), 'Report.pdf')
->withMime('application/pdf'),
];
}
}
Here, we require to create new controller PDFController that will manage index method of route. So let's put bellow code.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Mail\MailExample;
use PDF;
use Mail;
class PDFController extends Controller
{
/**
* Show the application dashboard.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$data["email"] = "[email protected]";
$data["title"] = "From shouts.dev";
$data["body"] = "This is Demo";
$pdf = PDF::loadView('emails.myTestMail', $data);
$data["pdf"] = $pdf;
Mail::to($data["email"])->send(new MailExample($data));
dd('Mail sent successfully');
}
}
In this is step we need to create routes for items listing. so open your "routes/web.php" file and add following route.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PDFController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('send-email-pdf', [PDFController::class, 'index']);
In Last step, let's create myTestMail.blade.php(resources/views/emails/myTestMail.blade.php) for layout of pdf file and put following code.
<!DOCTYPE html>
<html>
<head>
<title>shouts.dev</title>
</head>
<body>
<h1>{{ $title }}</h1>
<p>{{ $body }}</p>
<p>Thank you</p>
</body>
</html>
All the required steps have been done, now you have to type the given below command and hit enter to run the Laravel app.
php artisan serve
Now, Go to your web browser, type the given URL and view the app output.
http://localhost:8000/send-email-pdf
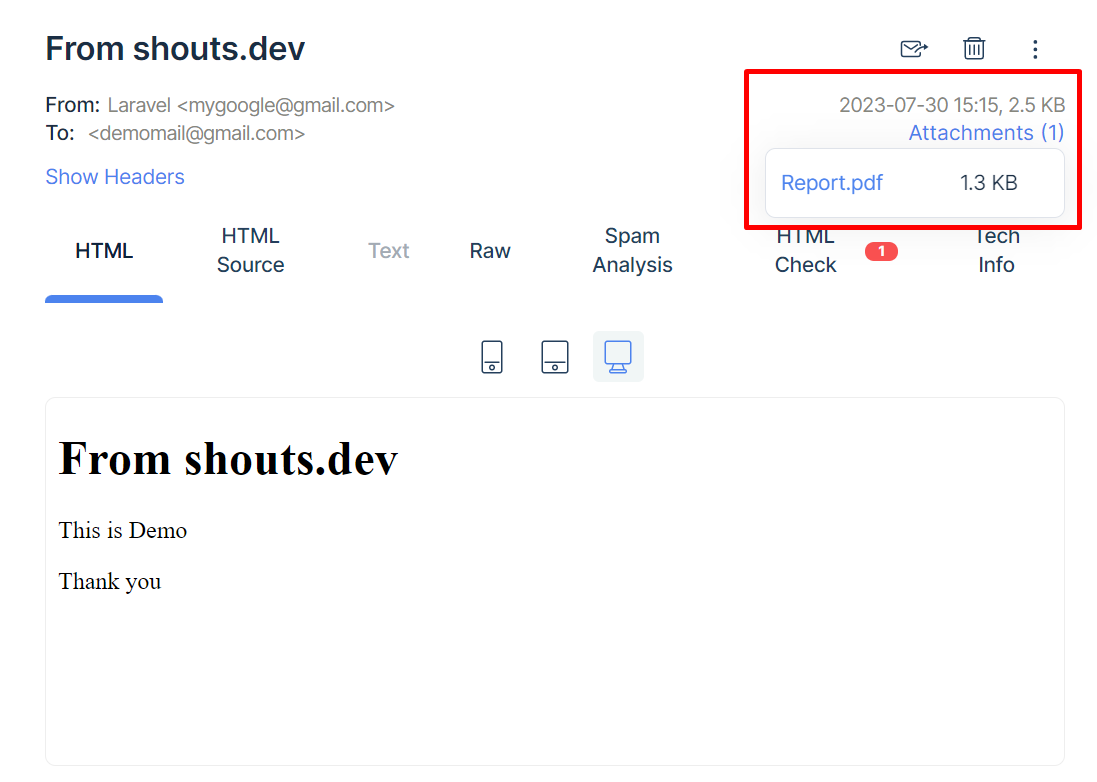
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. 🙂