Use Environment Variables in Laravel 9 & VueJs 3
Hello Artisans, today I'll show you how to use laravel environment variables in your vuejs app. Sometimes it's quite needy to access .env variables into our app.js or any .vue files. So, let's see how we can easily use .env variables into vue component or .js files.
Note: Tested on Laravel 9.11
Table of Contents
Add Variable to .env file
At first, we'll add a variable to our .env file and later we'll access this variable into our app.js file
VITE_APP_NAME="your_custom_name"
Install and Configure Vue and its Dependencies
For configure vue, at first, we'll install npm by firing the below command.
npm install
Then we'll install vue and vue loader so that our compiler can read vue template files.
npm install vue@next vue-loader@next
It'll create the latest version of vue 3.
Now we'll install vitejs plugin by firing the below command.
npm i @vitejs/plugin-vue
Now we'll configure our vite.config.js. So, open the file and replace with below codes
import { defineConfig } from 'vite';
import laravel from 'laravel-vite-plugin';
import vue from '@vitejs/plugin-vue'
export default defineConfig({
plugins: [
vue(),
laravel({
input: ['resources/css/app.css', 'resources/js/app.js'],
refresh: true,
}),
]
});
Now we'll create a component file under resources/js and put the below codes.
<template>
<div>
<h1>Hello Vue 3 App</h1>
</div>
</template>
<script>
export default {
name: "App"
}
</script>
<style scoped>
</style>
Now we'll modify our app.js file. Here we'll mount our app and we'll read the .env variable which we created at step1. So, replace your file with mine.
import './bootstrap';
import {createApp} from 'vue'
import App from './App.vue'
const appName = import.meta.env.VITE_APP_NAME;
alert(appName);
createApp(App).mount("#app")
Now run the below command to compile the files and start a development server
npm run dev
Now we'll complete with our vue setup. Now we'll go with our laravel setup.
Setup blade File
Now we've to setup our blade file. For that we'll use laravel default welcome.blade.php. Let's open the file and replace it with below codes.
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel</title>
</head>
<body>
<div id="app"></div>
@vite('resources/js/app.js')
</body>
</html>
Output
And finally, we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well, you'll find a below output.
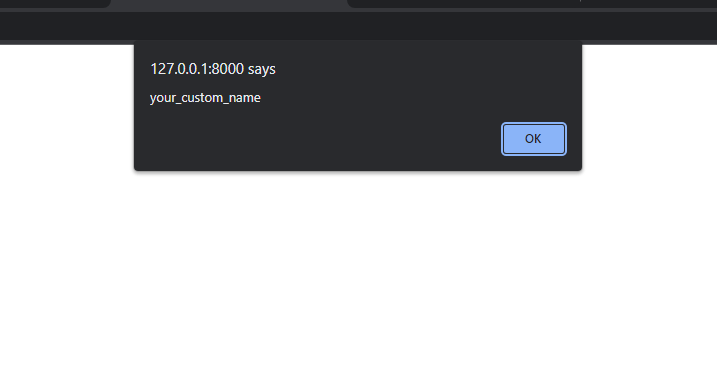
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐