How to Limit Login Attempts in Laravel
In this article, I’m going to show how to set limit login attempts. If user tries to log in more than 5 times per minute, they will get an error message. We’re able to customize the limit and block time. I’m testing on Laravel 7.17.2.
Table of Contents
Set Limit
Open app/Http/Controllers/Auth/LoginController.php file and add these 2 properties:
class LoginController extends Controller
{
protected $maxAttempts = 3; // default is 5
protected $decayMinutes = 2; // default is 1
// ...
}
Now if we enter 3 times wrong email or password in a row, we’ll see a warning message like:
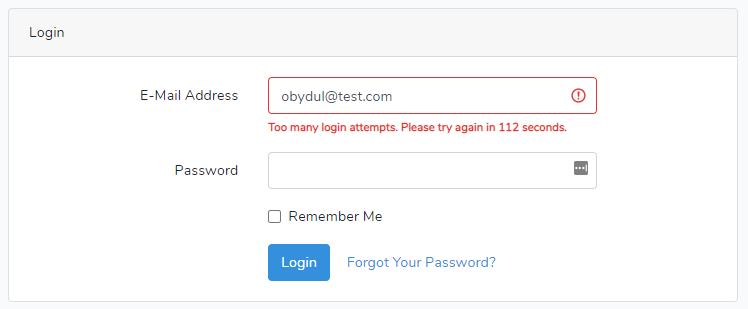
Change Error Text
We can change the error message easily. Open resources/lang/en/auth.php file and write your messages here:
return [
'failed' => 'These credentials do not match our records.',
'throttle' => 'Too many login attempts. Please try again in :seconds seconds.',
];
That’s it. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.