Integrate Google Firebase in Laravel
Firebase is a Backend-as-a-Service — BaaS — that started as a YC11 startup and grew up into a next-generation app-development platform on Google Cloud Platform.
In this article, I’m going to share how to integrate Google Firebase in Laravel application. Let’s get started:
Table of Contents
Create Firebase Project
Go to Firebase and create a project. After creating the project, go to the Setting->Project Settings->Service accounts. We need to download the Firebase Admin SDK configuration file.
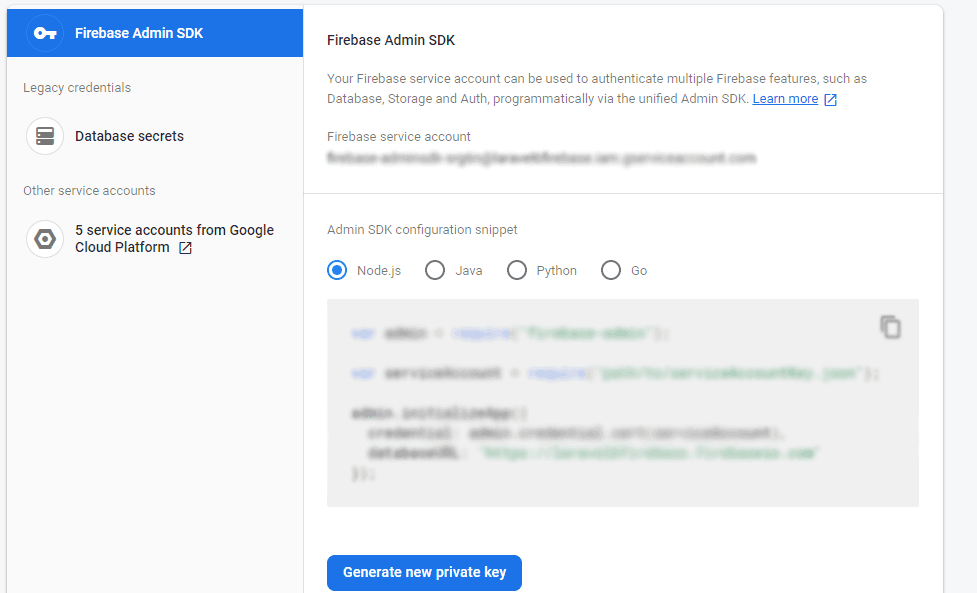
Click “Generate new private key” and it will download in a JSON file. Rename the file as firebase_credentials.json.
Install Package
Open Laravel application in terminal and install kreait/laravel-firebase package:
composer require kreait/laravel-firebase
Move the firebase_credentials.json file to the public folder of you app. Open .env file and addFIREBASE_CREDENTIALS
variable like this:
FIREBASE_CREDENTIALS=/path/public/firebase_credentials.json
I’m working on localhost and the file path: E:\laragon\www\laravel\public\firebase_credentials.json
Note: You can use config file too. Please read this configuration.
Create Controller
Let’s create a controller named FirebaseDBController:
php artisan make:controller FirebaseDBController
Open the controller from app\Http\Controllers folder and paste this code:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FirebaseDBController extends Controller
{
private $database;
public function __construct() {
$this->database = app('firebase.database');
}
/**
* Insert data.
*/
public function insert() {
$newPost = $this->database
->getReference('blog/posts')
->push([
'title' => "Title of Article",
'description' => "This is an article"
]);
return response()->json($newPost->getvalue());
}
/**
* Retrieve data.
*/
public function getData() {
$data = $this->database->getReference('blog/posts')->getvalue();
return response()->json($data);
}
/**
* Update data.
*/
public function update() {
$post_id = "-M56ibO-en8Z9O5ryzjK";
// new values
$postData = [
'title' => 'My awesome post title',
'description' => 'This text should be longer',
];
$updates = [
'blog/posts/'.$post_id => $postData,
];
$update = $this->database->getReference()->update($updates);
return response()->json($update->getvalue());
}
/**
* Delete data.
*/
public function delete() {
$post_id = "-M56jWfY-f7mHJYc5MtL";
$delete = $this->database->getReference('blog/posts/'.$post_id)->remove();
}
/**
* Delete all data.
*/
public function deleteAll() {
$delete = $this->database->getReference('blog/posts')->remove();
}
}
I’ve written CURD functions to store/delete data from realtime firebase database.
Define Routes
Let’s create some routes for the FirebaseDBController.
Route::prefix('firebase')->group(function () {
Route::get('insert', 'FirebaseDBController@insert');
Route::get('get_data', 'FirebaseDBController@getData');
Route::get('update', 'FirebaseDBController@update');
Route::get('delete', 'FirebaseDBController@delete');
Route::get('delete_all', 'FirebaseDBController@deleteAll');
});
Run and Test
Now run the project and visit the routes to check data is saving/deleting from realtime database or not.
I’ve visited http://example.test/firebase/insert
and checked realtime database from firebase console:
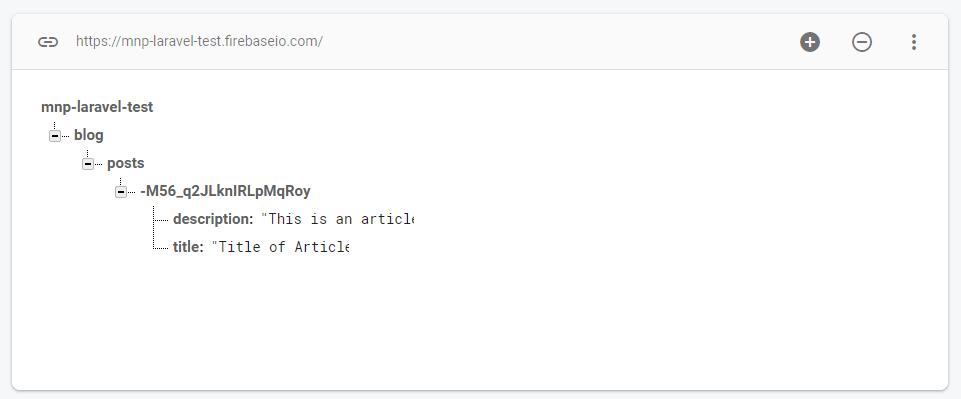
So, firebase is connected to our Laravel app & realtime database is working. Like the realtime database, now you can test FCM, Dynamic Links, Auth etc. Just take a look at laravel-firebase’s documentation.
The article is over. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.