Install Botman Chatbot in Laravel
Hello artisans,
Today I’ll show you how you can set up Botman Chatbot in your Laravel app easily. Botman chatbot is a kind of framework where you can use the bots for multiple messaging platforms, including Slack, Telegram, Microsoft Bot Framework, Nexmo, HipChat, Facebook Messenger, WeChat and many more. So, let’s see how we can implement the chatbot in our application.
Table of Contents
- Install Botman and Botman Driver
- Create Configuration File for Botman
- Create and Setup Controller
- Create Route
- Modify blade file
Install Botman and Botman Driver
To install the Botman you just need to fire the below command
composer require botman/botman
And to install the Botman Driver fire the blow command
composer require botman/driver-web
Create Configuration File for Botman
This step is not mandatory so you can skip without hesitation. But for better performance create a directory under config\botman and create two files config.php and web.php and finally paste the following code.
config\botman\config.php
<?php
return [
'conversation_cache_time' => 40,
'user_cache_time' => 30,
];
config\botman\web.php
<?php
return [
'matchingData' => [
'driver' => 'web',
],
];
Create and Setup Controller
At first, we need to create a controller so fire the below command to create a fresh controller.
php artisan make:controller BotmanController
This will create a file under App\Http\Controllers, open the file and paste the following code
<?php
namespace App\Http\Controllers;
use BotMan\BotMan\Messages\Incoming\Answer;
class BotmanController extends Controller
{
public function handle()
{
$botman = app('botman');
$botman->hears('{message}', function($botman, $message) {
if ($message == 'hi') {
$this->askName($botman);
}else{
$botman->reply("write 'hi' for testing...");
}
});
$botman->listen();
}
public function askName($botman)
{
$botman->ask('Hello! What is your Name?', function(Answer $answer) {
$name = $answer->getText();
$this->say('Nice to meet you '.$name);
});
}
}
Create Route
Put the following route in your web.php.
Route::match(['get', 'post'], '/botman', [\App\Http\Controllers\BotmanController::class,'handle']);
Modify blade file
We reached the final step of our tutorial. here we need to modify our welcome.blade.php a little bit. So, paste the following code in your blade file
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>How to install Botman Chatbot in Laravel? - shouts.dev</title>
<link href="https://fonts.googleapis.com/css?family=Nunito:200,600" rel="stylesheet">
</head>
<body>
</body>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/botman-web-widget@0/build/assets/css/chat.min.css">
<script>
var botmanWidget = {
aboutText: 'write something here',
introMessage: "✋ Hi! I'm form shouts.dev"
};
</script>
<script src='https://cdn.jsdelivr.net/npm/botman-web-widget@0/build/js/widget.js'></script>
</html>
And that’s it. You’ve successfully implemented botman in your application. now if you run your app you will see the below output
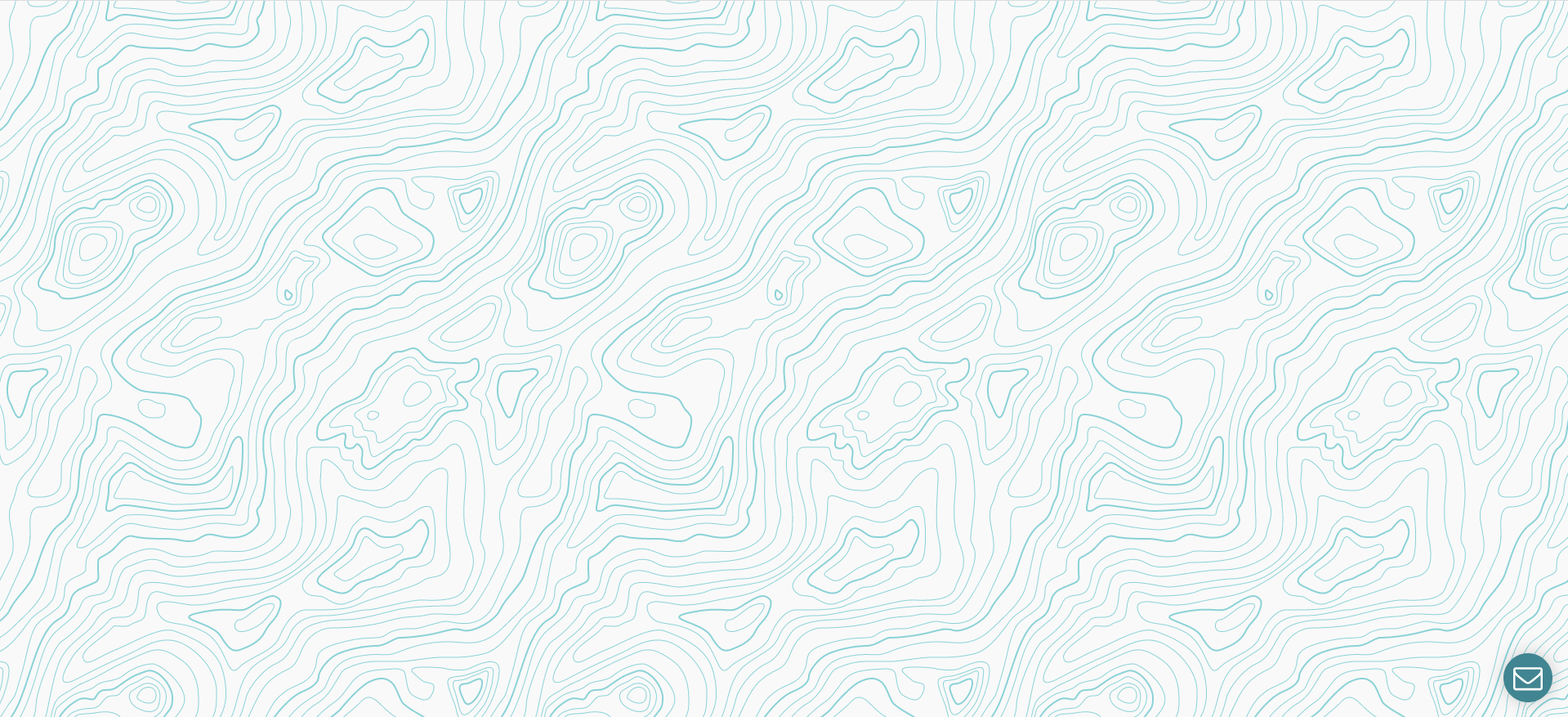
That’s all for toady. You can download this project from Github also. Thanks for reading.