JavaScript Create a Dropdown List using Array/Object
Hello dev's, today I'll show you how to create a dropdown list using JavaScript array/object. I'll give you two example of an array and with an object from which you can easily create a dropdown list. Let's see the examples
Example 1: Using array
In the first example we'll create a dropdown using array. Let's see the below code
<!DOCTYPE html>
<html>
<head>
<title>Create Dropdown usign Javascript - shouts.dev</title>
</head>
<body>
<div onclick="createDropdown()">
<button>Create Dropdown</button>
</div>
<p id="msg"></p>
<select id="select"></select>
<script>
var msg_area = document.getElementById('msg');
var select = document.getElementById("select");
var elmts = ["HTML", "CSS", "JS", "PHP", "Laravel","VueJs"];
// Main function
function createDropdown() {
for (var i = 0; i < elmts.length; i++) {
var optn = elmts[i];
var el = document.createElement("option");
el.textContent = optn;
el.value = optn;
select.appendChild(el);
}
msg_area.innerHTML = "Dropdown Created";
}
</script>
</body>
</html>
It produce the below output
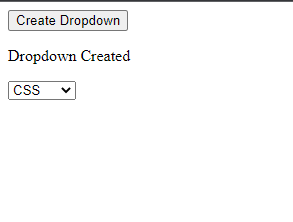
Example 2: Using object
In the below example we'll create a dropdown using object. Let's see the below code.
<!DOCTYPE html>
<html>
<head>
<title>Create Dropdown usign Javascript - shouts.dev</title>
</head>
<body>
<div onclick="createDropdown()">
<button>Create Dropdown</button>
</div>
<p id="msg"></p>
<select id="select"></select>
<script>
var msg_area = document.getElementById('msg');
var select = document.getElementById("select");
var elmts = [
{
id : 1,
title : "HTML"
},
{
id : 2,
title : "CSS"
},
{
id : 3,
title :"JS"
},
{
id : 4,
title :"PHP"
},
{
id : 5,
title :"Laravel"
},
{
id : 6,
title :"VueJs"
},
];
// Main function
function createDropdown() {
let length = Object.keys(elmts).length;
for (var i = 0; i < length; i++) {
var optn = elmts[i];
var el = document.createElement("option");
el.textContent = optn.title;
el.value = optn.id;
select.appendChild(el);
}
msg_area.innerHTML = "Dropdown Created";
}
</script>
</body>
</html>
Which will produce the same result which we see in the step1.
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐