How to Use map() in React Application
In react map is one of the most poplar and widely used function. It has two prominent use cases. Today we will see how to modify the state of the application and how to render a list of elements efficiently.
Let's get started.
In the following example, we will see how to manipulate arrays of data with map() efficiently.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
const doubled = numbers.map(number => number * 2)
// Log to console
console.log(doubled)
// in our console [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
The result of calling map() on an array will be a new array. Every element of this array is processed by the callback function provided to the map itself. So when we call map function on our array it takes each element and multiplies it by 2 and writes it out to the new array doubled.
Let’s take a look at another example. This will be a little bit complicated. We will use destructuring assignment to help us map over the list of objects and extract some data from them.
As you can see we have an array students. Each student is represented by an object. Each object has 3 properties, those are: id, name, and age. The goal here is to get an array of of students’ names and store it as an array.
The simplest way to carry out this operation is by using the map function.
const students = [
{ id: 1, name: 'Foe', age: 20},
{ id: 2, name: 'Bar', age: 25},
{ id: 3, name: 'Jon', age: 35}
];
const studentNames = students.map(({ name }) => name)
console.log(studentNames)
//in our console ["Foe", "Bar", "Jon"]
The callback functions returns the name, which gets written into the new array. And just like that we have an array of students’ names ready to use.
As we got the names of our students on the previous example, now we can map over them and render the names to the screen. Let's do this in our JSX now:
import React from 'react';
export function App(props) {
const studentNames = ["Foe", "Bar", "Jon"]
return (
<div className='App'>
<h1>Student's List</h1>
{studentNames.map((name, index) =>
<li key={index}> {name} </li>
)}
</div>
);
}
And here is our rendered output:
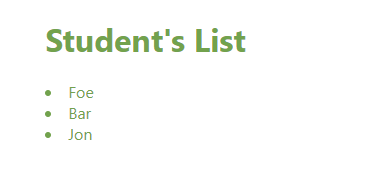
Always remember, each child in a list should have a unique key prop. Ignoring this can lead to some unwanted behavior in the future. That's why we use index number as the key.
Feel free to play around with mapping yourself. It’s a tool that every React developer should be confident with.
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading.