Easiest Way to Paginate an Array in JavaScript
Today I’m going to re-share one of the easiest ways to paginate array in JavaScript. I’ve found the method in arjun‘s blog. I like this simplest one.
Create an Array
Let’s create a simple product array. We’ll paginate this array.
let products = [
{
"id": 1,
"name": "Product 1",
},
{
"id": 2,
"name": "Product 2",
},
{
"id": 3,
"name": "Product 3",
},
{
"id": 4,
"name": "Product 4",
},
{
"id": 5,
"name": "Product 5",
},
{
"id": 6,
"name": "Product 6",
},
];
Create Paginator Function
Let’s create a paginator function. Here’s the function:
function paginator(items, current_page, per_page_items) {
let page = current_page || 1,
per_page = per_page_items || 10,
offset = (page - 1) * per_page,
paginatedItems = items.slice(offset).slice(0, per_page_items),
total_pages = Math.ceil(items.length / per_page);
return {
page: page,
per_page: per_page,
pre_page: page - 1 ? page - 1 : null,
next_page: (total_pages > page) ? page + 1 : null,
total: items.length,
total_pages: total_pages,
data: paginatedItems
};
}
Call Paginator Function
Now just call the function like this:
console.log(paginator(products, 2, 2));
Here’s the console log:
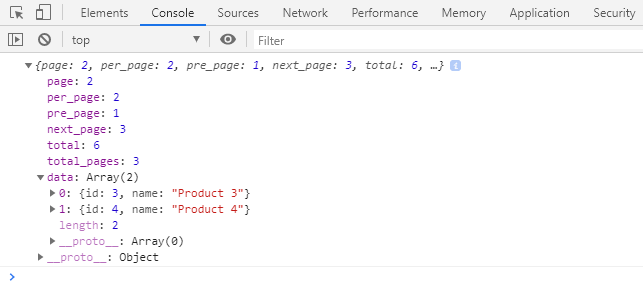
You can also call only call the function like paginator(products, 3)
. At this time, the next page will null. You can call in this way to get only one page data.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.