Node.js File System Module Example
Common use for the File System module:
- Read files
- Create files
- Update files
- Delete files
- Rename files
Node.js as a file server
Here is a Fs module code
const fs = require('fs');
First, we created a folder, inside the folder we are created the file name acme.js inside the root folder.
touch acme.js
We will delete this file using the fs module. So type the following code inside the acme.js file.
const fs = require('fs');
fs.unlink('acme.js', (err) => {
if (err) throw err;
console.log('File system operation successfully executed');
});
Run the following command in your terminal:
touch acme.js
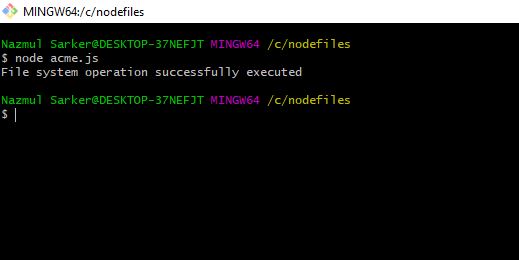
Using try-catch block
You can wrap the above operation in the try-catch block. Let us say we have already deleted the file and again we try to run the command then we will get the following error.
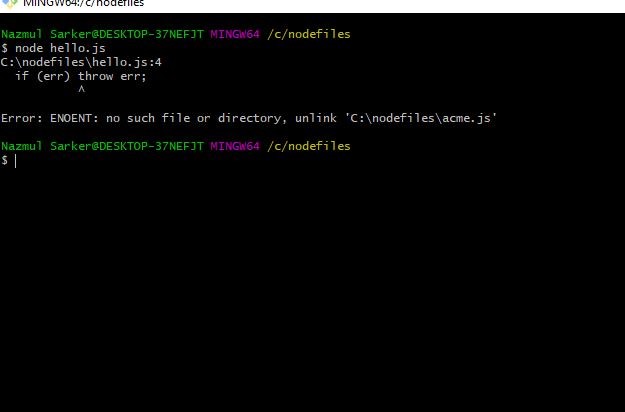
So, we need to define the try-catch error mechanism. Also for that, we need to perform the sync operation and not async operation.
const fs = require('fs');
try {
fs.unlinkSync('acme.js');
console.log('File system operation successfully executed');
}
catch(e) {
console.log('Excecuted Catch block');
console.log(e);
}
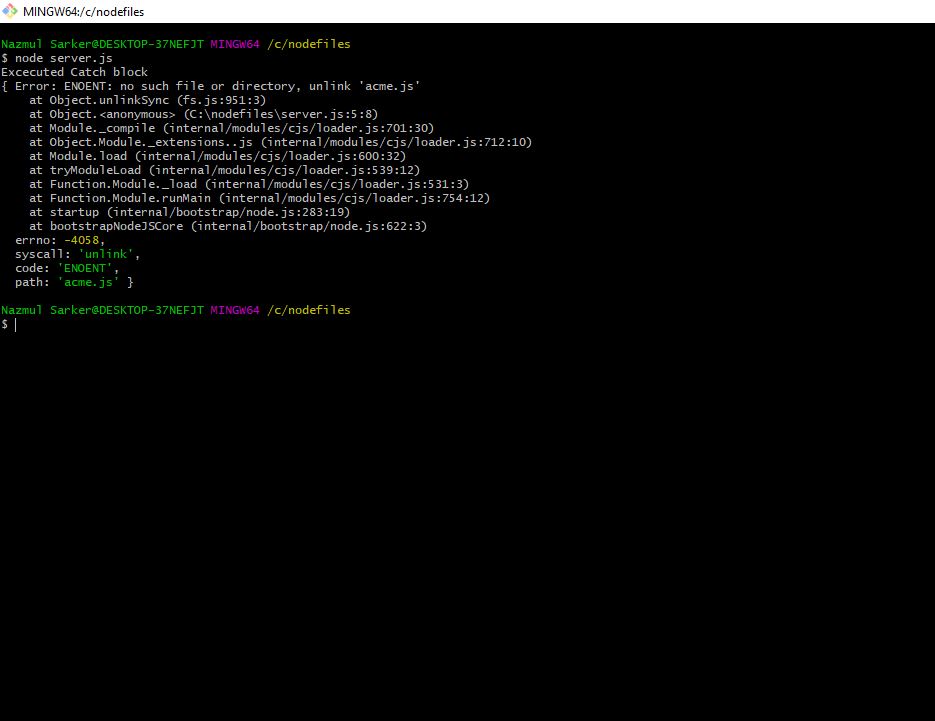
File paths
Most fs operations accept file paths that may be specified in the form of a string, a Buffer, or a URL object using the file protocol. String form paths are interpreted as UTF-8 character sequences identifying the absolute or relative filename. Relative paths will be resolved relative to the current working directory as specified by the process.cwd(). Recreate the acme.js file inside the root. Write the following code inside the server file.
const fs = require('fs');
fs.open('acme.js', 'r', (err, fd) => {
if (err) throw err;
console.log('File has opened');
fs.close(fd, (err) => {
console.log('File is closing');
if (err) throw err;
});
});
URL object support in node fs module
For most fs module functions, the path or filename argument may be passed as a WHATWG URL object. Only URL objects using the file protocol are supported.
const fs = require('fs');
const fileUrl = new URL('file:///acme.js');
fs.readFileSync(fileUrl);
Open a File using Node fs module
The syntax is following to open a file using node fs module.
fs.open(path, flags[, mode], callback)
Flags
Flags for reading/writing operations are the following.
Flag | Description |
---|---|
r | Open a file for reading. An exception occurs if a file does not exist. |
r+ | Open a file for reading and writing. An exception occurs if the file does not exist. |
rs | Open a file for reading in synchronous mode. |
rs+ | Open a file for reading and writing, asking the OS to open it synchronously. See notes for ‘rs’ about using this with caution. |
w | Open a file for writing. The file is created (if it does not exist) or truncated (if it exists). |
wx | Like ‘w’ but fails if the path exists. |
w+ | Open a file for reading and writing. The file is created (if it does not exist) or truncated (if it exists). |
wx+ | Like ‘w+’ but fails if the path exists. |
a | Open a file for appending. The file is created if it does not exist. |
ax | Like ‘a’ but fails if the path exists. |
a+ | Open file for reading and appending. The file is created if it does not exist. |
ax+ | Like ‘a+’ but fails if the path exists. |
Let us take an example of opening a file using the node fs module.
const fs = require('fs');
fs.open('acme.js', 'r+', function(err, fd) {
if (err) {
return console.error(err);
}
console.log("File opened successfully!");
});
Get File Information
Following is the syntax of the method to get the information about a particular file
fs.stat(path, callback)
Let us take an example of node file stat
const fs = require('fs');
fs.stat('acme.js', function (err, stats) {
if (err) {
return console.error(err);
}
console.log(stats);
console.log("Got file info successfully!");
// Check file type
console.log("isFile ? " + stats.isFile());
console.log("isDirectory ? " + stats.isDirectory());
});
Run and see the output in the terminal
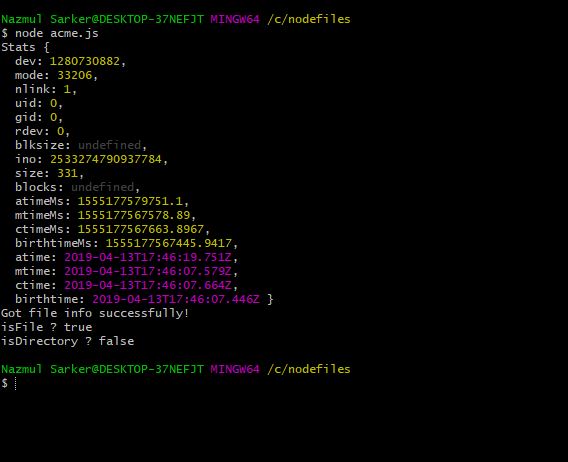
Writing a File using Node fs module
The syntax is following to write a file in node.js
fs.writeFile(filename, data[, options], callback
This method will overwrite the file if a file already exists. If you want to write into an existing file, then you should use another available method.
const fs = require('fs');
fs.writeFile('acme.js', 'console.log("hello")', function (err) {
if (err) throw err;
console.log('New file acme.js is either created or if exists then updated');
});
Reading a file using node fs module
To read a file in node.js, you can use the following syntax
fs.read(fd, buffer, offset, length, position, callback)
Let us take an example and understand how we can read the file using the node fs.
const http = require('http');
const fs = require('fs');
http.createServer(function (req, res) {
fs.readFile('acme.js', function(err, data) {
res.writeHead(200, {'Content-Type': 'text/js'});
res.write(data);
res.end();
});
}).listen(8080);
The above code will spin up the HTTP server and return the content of the file in the webpage. You can access the webpage at this URL: http://localhost:8080
Rename File
To rename a file with the File System module, use the fs.rename() method. The fs.rename() method renames the specified file.
const fs = require('fs');
fs.rename('filename.txt', 'filename2.txt', function (err) {
if (err) throw err;
console.log('File Renamed!');
});
Delete File
To delete a file with the File System module, use the fs.unlink() method. The fs.unlink() method deletes the specified file
const fs = require('fs');
fs.unlink('filename.txt', function (err) {
if (err) throw err;
console.log('File deleted!');
});
Run the file and the file will be removed from the disk.
Finally, Node js File System Module Example Tutorial is over.