Async-Await in Node.js
Async language constructs have been around in other languages for a while, like async/await in C#, coroutines in Kotlin and goroutines in Go. With the release of Node.js version 8, the long-awaited async functions have landed in Node.js as well.
What is Async/Await?
- It is the newest way to write asynchronous code in JavaScript.
- It is non-blocking (just like callbacks and promises).
- Async/Await is created to simplify the process of working with and writing chained promises.
Syntax of Async Function
function add(a,b){
return a + b;
}
// Async Function
async function add(a,b){
return a + b;
}
The only fundamental difference is that they always yield a Promise instead of a { value: any, done: boolean } object.
Now, create a project folder
mkdir node-examples
Go into the project folder
cd node-examples
Now, create a package.json file using the following command
npm init -y
Install the nodemon server using the following command
npm install nodemon
Create a new file called server.js inside the root.
function square(x) {
return new Promise(resolve => {
setTimeout(() => {
resolve(Math.pow(x, 2));
}, 2000);
});
}
square(10).then(data => {
console.log(data);
});
Next step is to start the nodemon server using the following command
nodemon server
So, here after two seconds, we can see the square of 10. Function square returns the promise, and after resolving that promise, we get our squared data.Switching from Promises to Asyn
Switching from Promises to Async/Await
now turn above code into async/await function
function square(x) {
return new Promise(resolve => {
setTimeout(() => {
resolve(Math.pow(x, 2));
}, 2000);
});
}
async function layer(x)
{
const value = await square(x);
console.log(value);
}
layer(10);
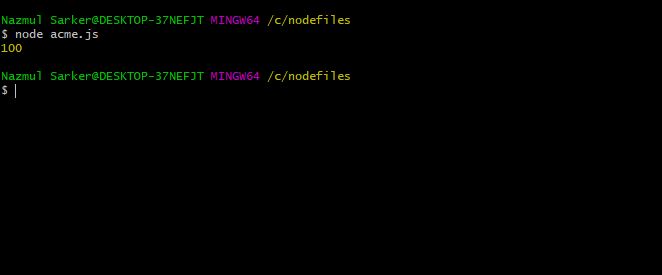
Async/Await in AJAX Request
First, we need to install the node.js client specific library to send an ajax request. We use the node-fetch library.
npm install node-fetch --save
For the ajax request, we can use the async-await function like the following
const fetch = require('node-fetch');
async function asyncajaxawait(x)
{
const res = await fetch('https://api.github.com/users/mdobydullah')
const data = await res.json();
console.log(data.name);
}
asyncajaxawait(10);
Here, we have used the node-fetch library to send an ajax request to the Github API and get the response.