Laravel Get Current User Location by IP
Hello Artisans, today I'll show you how to get current location by user client IP. Sometimes we need to check user IP for our security reason and we only wants to allow only the specific one IP. So, no more talk let's see how we can easily take the user location based on their IP address.
Note: Tested on Laravel 9.19.
Table of Contents
- Install stevebauman/location package
- Create and Setup Controller
- Create and Setup Route
- Create and Setup View File
- Output
Install stevebauman/location package
We'll use a package called stevebauman/location, this package is very handy and easy to use. You can find more information here. So let's fire the below command in terminal.
composer require stevebauman/location
And that's it we don't need to do anything. Let's go for next step.
Create and Setup Controller
First of all, we'll create a controller so that we can write our desire logic to get the location. So, fire the below commands in the terminal.
php artisan make:controller UserController
It'll create a file under app\Http\Controllers called UserController.php. Open the file and replace with below codes.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Stevebauman\Location\Facades\Location;
class UserController extends Controller
{
public function index()
{
$ip = '106.0.52.138'; /* Static IP address */
//use your own ip address
$data = [
'location' => Location::get($ip)
];
return view('location', $data);
}
}
Create and Setup Route
Let's put the below route in web.php
Route::get('get-location', [UserController::class, 'index'])->name('get.location');
resources/views/location.blade.php
Now we we'll create a blade file where we can setup our view where we can see the user location details based on their IP. So let's create a view file called location.blade.php under resources/views and paste the below code in the following file.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h2 class="text-center mt-5 mb-3">How to Get Current User Location - shouts.dev</h2>
<div class="card">
<div class="card-body">
@if($location)
<table class="table table-hover table-striped">
<thead>
<tr>
<td>Property</td>
<td>Value</td>
</tr>
</thead>
<tbody>
@foreach($location as $key => $item)
@if($item)
<tr>
<td class="text-capitalize">{{ $key }}</td>
<td>{{ $item }}</td>
</tr>
@endif
@endforeach
</tbody>
</table>
@endif
</div>
</div>
</div>
</body>
</html>
Output
And finally we're ready with our setup. It's time to check our output. Now go to http://localhost:8000/get-location, If everything goes well (hope so) you can find a below output on.
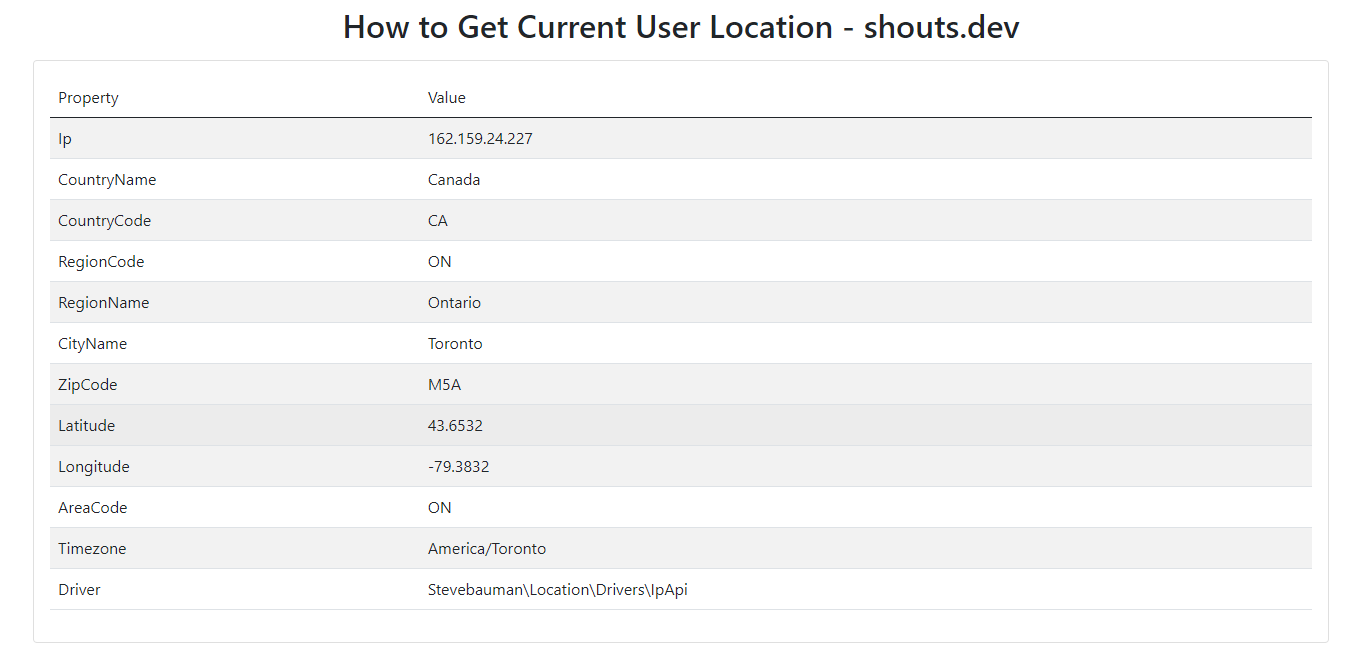
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. 🙂