Laravel Eloquent One to One Relationship Tutorial with Example
A one-to-one relationship is a very basic relation. In this guide, I’m going to explain one to one eloquent relationship model. I’ll show how to insert data and retrieve data using the eloquent model.
In this tutorial, I’ll create two tables called users and mobile. Here’s the schema design:
Note: This article last tested on Laravel 7.x. But it’ll work on Laravel 8.x too. Just define models in app/Models
folder. That’s it.
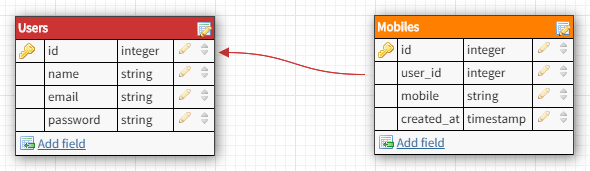
Table of Contents
- Install Laravel and Basic Configurations
- Create Migration and Model
- Setup One To One Relationship
- Inverse Of The Relationship
- Insert Records
- Retrieve Records
- Update Records
- Delete Records
Step 1 : Install Laravel and Basic Configurations
Each Laravel project needs this thing. That’s why I have written an article on this topic. Please see this part from here: Install Laravel and Basic Configurations.
Step 2 : Create Migration and Model
We have already the User model and migration file. Let’s create a model named Mobile with a migration file. Run this command to create both:
php artisan make:model Mobile -m
Now open the migration file for the Mobile model from database>migrations
directory. Then paste the below code in the up()
function of the migration file.
public function up()
{
Schema::create('mobiles', function (Blueprint $table) {
$table->bigIncrements('id');
$table->unsignedBigInteger('user_id');
$table->string('mobile');
$table->timestamps();
$table->foreign('user_id')->references('id')->on('users')
->onDelete('cascade');
});
}
We have set user_id as a foreign key and mobile number will be deleted if we delete the user.
Step 3 : Setup One To One Relationship
We have two models User & Mobile. For example, a User
model might be associated with one Mobile
. To define this relationship, we place a mobile
method on the User
model. The mobile
method should call the hasOne
method and return its result.
<?php
namespace App;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
// ...
/**
* Get the mobile number associated with the user.
*/
public function mobile()
{
return $this->hasOne(Mobile::class);
// note: we can also inlcude Mobile model like: 'App\Mobile'
}
}
Step 4 : Inverse Of The Relationship
So, we can access the Mobile
model from our User
. Now, let’s define a relationship on the Mobile
model that will let us access the User
that owns the phone. We can define the inverse of a hasOne
relationship using the belongsTo
method.
Open Mobile model and paste this code:
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Mobile extends Model
{
/**
* Get the user that owns the mobile.
*/
public function user()
{
return $this->belongsTo(User::class);
}
}
Step 5 : Insert Records
Let’s add data to user and mobile from controller:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\User;
use App\Mobile;
use Hash;
class UserController extends Controller
{
public function addUserMobile()
{
$user = new User;
$user->name = "Test Name";
$user->email = "[email protected]";
$user->password = Hash::make("12345678");
$user->save();
$mobile = new Mobile;
$mobile->mobile = '123456789';
$user->mobile()->save($mobile);
}
}
Step 6 : Retrieve Records
We can easily get data from relation model like this:
public function index()
{
// get user and mobile data from User model
$user = User::find(1);
var_dump($user->name);
var_dump($user->mobile->mobile);
// get user data from Mobile model
$user = Mobile::find(1)->user;
dd($user);
// get mobile number from User model
$mobile = User::find(1)->mobile;
dd($mobile);
}
Step 7: Update Records
Let’s update the user’s table data and mobile’s table data at once:
public function update()
{
$user = User::find(1);
$user->name = 'Test II';
$user->mobile->mobile = '987654321';
$user->push();
}
Step 8 : Delete Records
We can easily delete both table’s data at once. Here’s the example:
public function delete()
{
$user = User::find(1);
$user->delete();
}
To get more details of one to one eloquent relationship, you can read Laravel’s official documentation.
The tutorial is over. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.