Add or Remove Input Fields Dynamically using jQuery
Today I’m going to share how to make dynamic input fields using jQuery with add, remove option. Let’s start:
Table of Contents
Step 1 : Include jQuery & Bootstrap
It is not necessary to include Bootstrap. I’m going to include Bootstrap to make a simple design. We will include jQuery & Bootstrap’s CDNs. Here are the CDN links:
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"> <script src="//ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
Step 2 : Create a Simple Form
Let’s create a simple form including one text field. We will make the text field dynamic:
<form method="post" action="">
<div class="row">
<div class="col-lg-12">
<div id="inputFormRow">
<div class="input-group mb-3">
<input type="text" name="title[]" class="form-control m-input" placeholder="Enter title" autocomplete="off">
<div class="input-group-append">
<button id="removeRow" type="button" class="btn btn-danger">Remove</button>
</div>
</div>
</div>
<div id="newRow"></div>
<button id="addRow" type="button" class="btn btn-info">Add Row</button>
</div>
</div>
</form>
Step 3 : Make Field Dynamic
We have created a simple form with one text field. We are going to make the text field dynamic using jQuery. Here’s the jQuery code:
<script type="text/javascript">
// add row
$("#addRow").click(function () {
var html = '';
html += '<div id="inputFormRow">';
html += '<div class="input-group mb-3">';
html += '<input type="text" name="title[]" class="form-control m-input" placeholder="Enter title" autocomplete="off">';
html += '<div class="input-group-append">';
html += '<button id="removeRow" type="button" class="btn btn-danger">Remove</button>';
html += '</div>';
html += '</div>';
$('#newRow').append(html);
});
// remove row
$(document).on('click', '#removeRow', function () {
$(this).closest('#inputFormRow').remove();
});
</script>
Step 4 : The Full Code
We have finished all the tasks. Here’s the full code:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Add or Remove Input Fields Dynamically using jQuery - MyNotePaper</title>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<script src="//ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
</head>
<body>
<div class="container"style="max-width: 700px;">
<div class="text-center" style="margin: 20px 0px 20px 0px;">
<a href="https://shouts.dev/" target="_blank"><img src="https://i.imgur.com/hHZjfUq.png"></a><br>
<span class="text-secondary">Add or Remove Input Fields Dynamically using jQuery</span>
</div>
<form method="post" action="">
<div class="row">
<div class="col-lg-12">
<div id="inputFormRow">
<div class="input-group mb-3">
<input type="text" name="title[]" class="form-control m-input" placeholder="Enter title" autocomplete="off">
<div class="input-group-append">
<button id="removeRow" type="button" class="btn btn-danger">Remove</button>
</div>
</div>
</div>
<div id="newRow"></div>
<button id="addRow" type="button" class="btn btn-info">Add Row</button>
</div>
</div>
</form>
</div>
<script type="text/javascript">
// add row
$("#addRow").click(function () {
var html = '';
html += '<div id="inputFormRow">';
html += '<div class="input-group mb-3">';
html += '<input type="text" name="title[]" class="form-control m-input" placeholder="Enter title" autocomplete="off">';
html += '<div class="input-group-append">';
html += '<button id="removeRow" type="button" class="btn btn-danger">Remove</button>';
html += '</div>';
html += '</div>';
$('#newRow').append(html);
});
// remove row
$(document).on('click', '#removeRow', function () {
$(this).closest('#inputFormRow').remove();
});
</script>
</body>
</html>
Step 5 : Run and See the Output
Now let’s run this file and see the output. I’ve run and here’s the output:
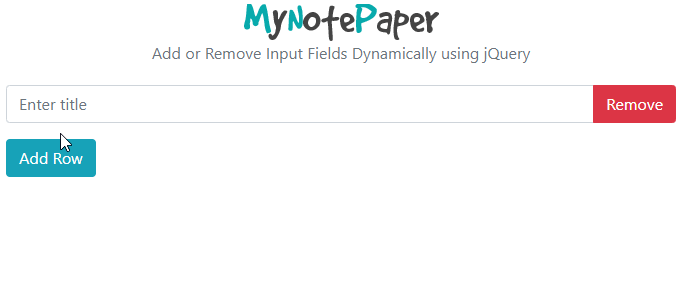
The topic is over. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.