Understanding OOP Concepts: Encapsulation
In this article, we’re going to learn about Encapsulation. I hope you’ll get the basic idea. Let’s get started:
Table of Contents
What is Encapsulation
Encapsulation a mechanism of wrapping data together into a single unit. Using Encapsulation, we mainly hide “sensitive” data from users. For example, a capsule that is mixed of several medicines.
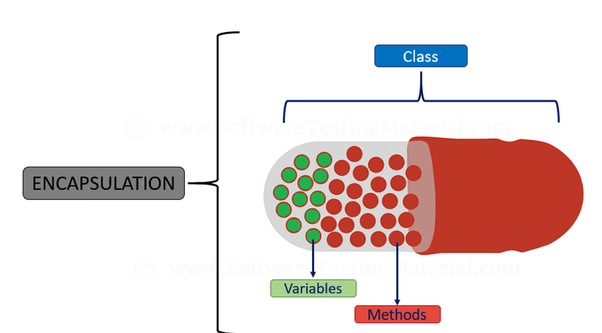
To achieve Encapsulation:
- Declare properties/attributes as
private
. - Use get and set methods to access and update the value of a
private
variables/properties.
Example in Java
Let’s see an example of Encapsulation:
public class Person {
private String name; // private access
// set
public void setName(String newName) {
this.name = newName;
}
// get
public String getName() {
return name;
}
}
We’ve defined name property as private. So, we’re unable to access this variable from outside of the class.
public class AnotherClass {
public static void main(String[] args) {
Person personObject = new Person();
personObject.name = "Obydul"; // will show error
System.out.println(personObject.name); // will show error
}
}
We’ve to use setter & getter methods to access or update values like this:
public class AnotherClass {
public static void main(String[] args) {
Person personObject = new Person();
personObject.setName("Obydul"); // set value
System.out.println(personObject.getName()); // get value
}
}
Advantages of Encapsulation
Let’s see some advantages of Encapsulation:
- Increased security of data by hiding important data.
- It provides the control of class attributes and methods.
- The attributes/variables of a class can be made read-only or write-only.
- Encapsulation is better for unit testing.
- It improves maintainability, flexibility and re-usability. Since the implementation is purely hidden for outside classes.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.