Laravel How to Add Toastr Notifications
Hello Artisans, today I'll talk about implementing Toastr notifications in our Laravel application. Toastr is JavaScript library which display the message in the form of notification. It can be success, warning, info or error. It is necessary when a user performs an action and we've to notify that user. So, let's see how we can user Toastr notifications in our application.
Note: Tested on Laravel 9.2.
Table of Contents
- Install brian2694/laravel-toastr
- Add CSS and JS to html
- Create and Setup Controller
- Define Route
- Output
Install brian2694/laravel-toastr
Firstly we need to install the brian2694/laravel-toastr package. Run the below command in your terminal to install :
composer require brian2694/laravel-toastr
Add CSS and JS to html
Now we will modify the Laravel default welcome.blade.php. So, put the below code in yours.
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel Toastr Notification</title>
<link rel="stylesheet" href="http://cdn.bootcss.com/toastr.js/latest/css/toastr.min.css">
</head>
<body class="antialiased">
<script src="http://cdn.bootcss.com/jquery/2.2.4/jquery.min.js"></script>
<script src="http://cdn.bootcss.com/toastr.js/latest/js/toastr.min.js"></script>
{!! Toastr::message() !!}
</body>
</html>
Create and Setup Controller
First we'll create a controller where we can use our logic to show user a toastr notification. Fire the below command in your terminal to create a controller.
php artisan make:controller ToastrNotificationController
Now put the below code in ToastrNotificationController.php.
<?php
namespace App\Http\Controllers;
use Brian2694\Toastr\Facades\Toastr;
class ToastrNotificationController extends Controller
{
public function toastrNotification()
{
//put your logic here
Toastr::success('You Have Successfully Implement Toastr Notification :)', 'Success!!');
return view('welcome');
}
}
Define Route
Now Replace your web.php file with the below codes.
<?php
use Illuminate\Support\Facades\Route;
Route::get('toastr-notification',[\App\Http\Controllers\ToastrNotificationController::class,'toastrNotification']);
Output
Now if you go to http://127.0.0.1:8000/toastr-notification you'll see the below notification in the top right most corner.
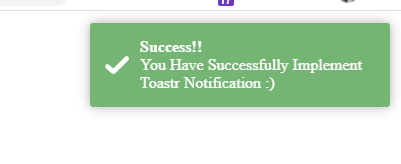
That's it for today. Hope you'll enjoy this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐