Understanding OOP Concepts: Properties and Methods
In part 1, we’ve learned about OPP, Class & Object. Today I’m trying to explain properties and methods. Let’s get started:
Table of Contents
Properties
A property is an attribute of an object. We can also say the variables inside a class are called properties.
Methods
A function inside a class is called a method. A method is an action that an object can perform.
Example
Let’s assume a Car class. I’m writing some possible properties and objects of the Car class.
Class: Car
Object: BMW i8, Tesla 3, Audi a3
Properties: Brand, Color, SunRoof
Methods: Horn, Steering
This figure helps us to get a clear idea:
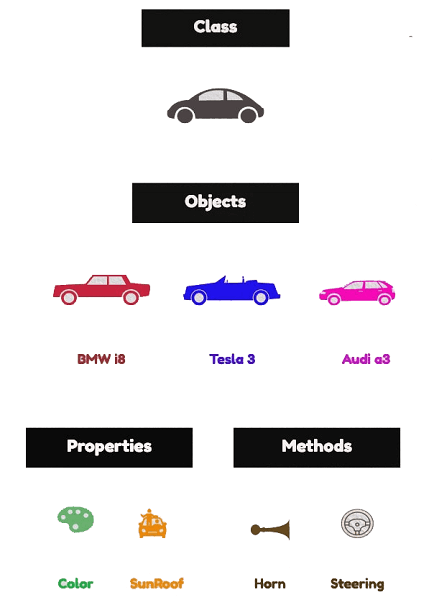
Example in Java
Now let’s try to write the code of Car class:
public class Car {
// properties
String brand;
String color;
Boolean hasSunRoof = true;
// method
void horn() {
System.out.println("Beep...!");
}
// method
void steering() {
System.out.println("Move the car...!\n");
}
// method
void displayInfo() {
String sunRoof;
if (hasSunRoof)
sunRoof = "has Sunroof";
else
sunRoof = "doesn't have Sunroof";
System.out.println("Car brand is " + brand + ". It's color is " + color + " and " + sunRoof);
}
}
We’ve written the Car class code. Let’s try to create objects and display information:
public class AnotherClass {
public static void main(String[] arg) {
// object bmw
Car bmw = new Car();
bmw.brand = "BMW i8";
bmw.color = "Black";
bmw.displayInfo();
bmw.horn();
bmw.steering();
// object audi
Car audi = new Car();
audi.brand = "Audi a3";
audi.color = "Red";
audi.displayInfo();
audi.horn();
audi.steering();
}
}
In the next article, I’ll try to explain constructor & destructor.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.