Node.js Connect MySQL Database with Example
We can easily connect to the MySQL database in Node.js. Node.js have a driver for MySQL. If you are very beginners in Node.js, please read this article first Build a Simple Node.js Web Server for Beginners
Table of Contents
Step 1 : Install MySQL
Navigate to your project folder and run this command to install MySQL:
npm install mysql
After successfully installation, you will see mysql folder under node_modules directory.
Step 2 : Create a Database
Let’s create a database named ‘test‘ and create a table named ‘users‘. After creating test database, you can run this SQL to create users table:
CREATE TABLE `users` (
`id` int(11) NOT NULL,
`name` varchar(50) COLLATE utf8_unicode_ci NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
Now import sample data to users table:
INSERT INTO `users` (`id`, `name`) VALUES
(1, 'Md Obydullah'),
(2, 'Md Najmul Hasan'),
(3, 'Md Mehadi Hasan');
Step 3 : Create & Run server.js File
Let’s create a js file named ‘server.js’ and paste this code:
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : '',
database : 'test'
});
connection.connect();
connection.query('SELECT * FROM users', function(err, result, fields)
{
if (err) throw err;
console.log(result);
});
connection.end();
Now we have to run this file by typing this command:
node server.js
Output:
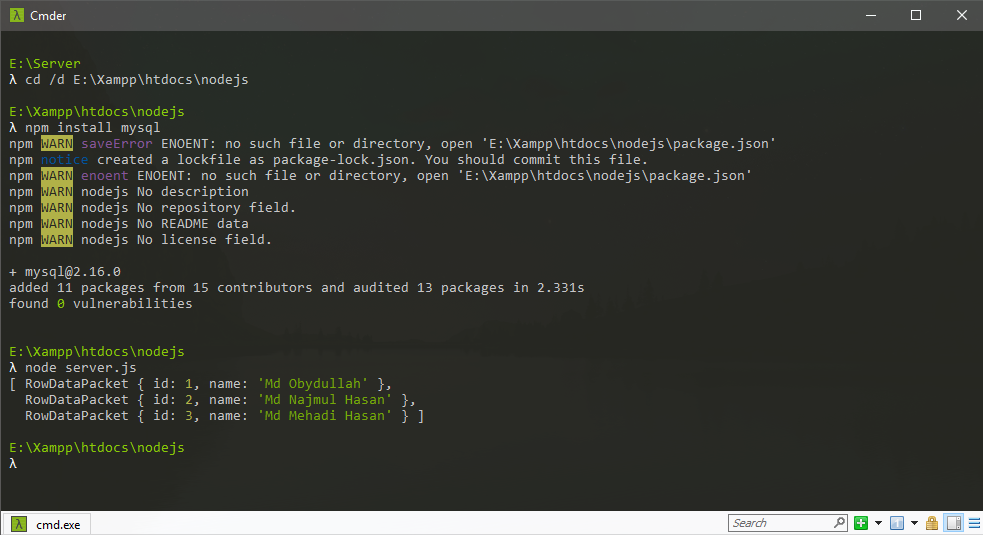
I hope this article will help you. ?
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.