Laravel SPA with Vue 3, Auth (Sanctum), CRUD Example
In this tutorial, we are going to create a single page application with Auth using Laravel and Vue 3. Let’s start:
Tested with: Laravel 8.25.0, Vue 3.
Table of Contents
- Install Laravel and NPM Dependencies
- Install Sanctum
- Create Migration, Model and Controller
- Define Laravel Routes
- Create Vue App
- Create Vue Pages
- Create Vue Components
- Define Vue Routes
- Import All to app.js
- The Output
Install Laravel and NPM Dependencies
Each Laravel project needs this thing. That’s why I have written an article on this topic. Please see this part from here: Install Laravel and Basic Configurations.
Now, we need to install latest vue, vue-router, compiler-sfc and vue-loader. Before installing these packages, remove vue
& vue-template-compiler
dependencies from package.json
.
Now let’s install the dependencies:
# dependencies
npm i vue@next vue-router@next
# devDependencies
npm i -D @vue/compiler-sfc vue-loader@next
After installing the dependencies run this command:
npm i && npm run dev
This npm run watch
command will listen for file changes and will compile assets instantly. The command npm run dev
won’t listen for file changes.
Install Sanctum
Laravel Sanctum provides a featherweight authentication system for SPAs (single page applications), mobile applications, and simple, token based APIs. Install sanctum:
composer require laravel/sanctum
Publish the configuration files and migrations:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Add Sanctum’s middleware to your api
middleware group within your application’s app/Http/Kernel.php
file:
'api' => [
\Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
Open up the config/sanctum.php
file and add your domain (mine: laravel.test) to this list:
'stateful' => explode(',', env(
'SANCTUM_STATEFUL_DOMAINS',
'localhost,localhost:3000,127.0.0.1,127.0.0.1:8000,::1,laravel.test'
)),
Open .env
, update your session driver to cookie.
SESSION_DRIVER=cookie
Lastly, open config/cors.php
config file and set the supports_credentials
option to true
.
'supports_credentials' => true
Create Migration, Model and Controller
We are going to create Book model and migration, API user & book controllers. Run these commands:
php artisan make:model Book -m
php artisan make:controller API/BookController
php artisan make:controller API/UserController
Now open create_books_table.php migration file from database>migrations and replace up() function with this:
public function up()
{
Schema::create('books', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('name');
$table->string('author');
$table->timestamps();
});
}
Migrate the database using the following command:
php artisan migrate
Open Book.php model from app/Models folder and paste this code:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Book extends Model
{
use HasFactory;
protected $fillable = ['name', 'author'];
}
We need to define the index, add, edit, delete methods in BookController file. Open BookController.php from app>Http>Controllers>API folder and paste this code:
<?php
namespace App\Http\Controllers\API;
use App\Http\Controllers\Controller;
use App\Models\Book;
use Illuminate\Http\Request;
class BookController extends Controller
{
// all books
public function index()
{
$books = Book::all()->toArray();
return array_reverse($books);
}
// add book
public function add(Request $request)
{
$book = new Book([
'name' => $request->name,
'author' => $request->author
]);
$book->save();
return response()->json('The book successfully added');
}
// edit book
public function edit($id)
{
$book = Book::find($id);
return response()->json($book);
}
// update book
public function update($id, Request $request)
{
$book = Book::find($id);
$book->update($request->all());
return response()->json('The book successfully updated');
}
// delete book
public function delete($id)
{
$book = Book::find($id);
$book->delete();
return response()->json('The book successfully deleted');
}
}
Now open UserController.php from the same path and paste:
<?php
namespace App\Http\Controllers\API;
use Session;
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Illuminate\Support\Facades\Hash;
use App\Http\Controllers\Controller;
class UserController extends Controller
{
/**
* Register
*/
public function register(Request $request)
{
try {
$user = new User();
$user->name = $request->name;
$user->email = $request->email;
$user->password = Hash::make($request->password);
$user->save();
$success = true;
$message = 'User register successfully';
} catch (\Illuminate\Database\QueryException $ex) {
$success = false;
$message = $ex->getMessage();
}
// response
$response = [
'success' => $success,
'message' => $message,
];
return response()->json($response);
}
/**
* Login
*/
public function login(Request $request)
{
$credentials = [
'email' => $request->email,
'password' => $request->password,
];
if (Auth::attempt($credentials)) {
$success = true;
$message = 'User login successfully';
} else {
$success = false;
$message = 'Unauthorised';
}
// response
$response = [
'success' => $success,
'message' => $message,
];
return response()->json($response);
}
/**
* Logout
*/
public function logout()
{
try {
Session::flush();
$success = true;
$message = 'Successfully logged out';
} catch (\Illuminate\Database\QueryException $ex) {
$success = false;
$message = $ex->getMessage();
}
// response
$response = [
'success' => $success,
'message' => $message,
];
return response()->json($response);
}
}
Define Laravel Routes
Our model, migration and controller are ready to use. Let’s define the web and API routes. Open web.php from routes folder and register this route:
<?php
use Illuminate\Support\Facades\Route;
Route::get('{any}', function () {
return view('app');
})->where('any', '.*');
Open api.php and define these routes:
<?php
use App\Http\Controllers\API\BookController;
use App\Http\Controllers\API\UserController;
use Illuminate\Support\Facades\Route;
Route::post('login', [UserController::class, 'login']);
Route::post('register', [UserController::class, 'register']);
Route::post('logout', [UserController::class, 'logout'])->middleware('auth:sanctum');
Route::group(['prefix' => 'books', 'middleware' => 'auth:sanctum'], function () {
Route::get('/', [BookController::class, 'index']);
Route::post('add', [BookController::class, 'add']);
Route::get('edit/{id}', [BookController::class, 'edit']);
Route::post('update/{id}', [BookController::class, 'update']);
Route::delete('delete/{id}', [BookController::class, 'delete']);
});
Create Vue App
To declaratively render data to the DOM using Vue.js we need to declare Vue app. Navigate to resources>views folder and create a file called app.blade.php. Then paste this code:
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" content="{{ csrf_token() }}"/>
<title>{{env('APP_NAME')}}</title>
<link href="{{ mix('css/app.css') }}" type="text/css" rel="stylesheet"/>
</head>
<body>
@if (Auth::check())
<script>
window.Laravel = {!!json_encode([
'isLoggedin' => true,
'user' => Auth::user()
])!!}
</script>
@else
<script>
window.Laravel = {!!json_encode([
'isLoggedin' => false
])!!}
</script>
@endif
<div id="app">
</div>
<script src="{{ mix('js/app.js') }}" type="text/javascript"></script>
</body>
</html>
NOTE: I’ve noticed many devs are facing an error with the previous app.blade.php’s code. If you are also face an error, please try with this code:
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" value="{{ csrf_token() }}"/>
<title>{{env('APP_NAME')}}</title>
<link href="{{ mix('css/app.css') }}" type="text/css" rel="stylesheet"/>
</head>
<body>
@if (Auth::check())
@php
$user_auth_data = [
'isLoggedin' => true,
'user' => Auth::user()
];
@endphp
@else
@php
$user_auth_data = [
'isLoggedin' => false
];
@endphp
@endif
<script>
window.Laravel = JSON.parse(atob('{{ base64_encode(json_encode($user_auth_data)) }}'));
</script>
<div id="app">
</div>
<script src="{{ mix('js/app.js') }}" type="text/javascript"></script>
</body>
</html>
Go to resources>js folder and create a file called App.vue and paste this code:
<template>
<div class="container">
<div class="text-center" style="margin: 20px 0px 20px 0px;">
<a href="https://shouts.dev/" target="_blank"><img src="https://i.imgur.com/Nt3kJXa.png"></a><br>
<span class="text-secondary">Laravel SPA with Vue 3, Auth (Sanctum), CURD Example</span>
</div>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<div class="collapse navbar-collapse">
<!-- for logged-in user-->
<div class="navbar-nav" v-if="isLoggedIn">
<router-link to="/dashboard" class="nav-item nav-link">Dashboard</router-link>
<router-link to="/books" class="nav-item nav-link">Books</router-link>
<a class="nav-item nav-link" style="cursor: pointer;" @click="logout">Logout</a>
</div>
<!-- for non-logged user-->
<div class="navbar-nav" v-else>
<router-link to="/" class="nav-item nav-link">Home</router-link>
<router-link to="/login" class="nav-item nav-link">login</router-link>
<router-link to="/register" class="nav-item nav-link">Register
</router-link>
<router-link to="/about" class="nav-item nav-link">About</router-link>
</div>
</div>
</nav>
<br/>
<router-view/>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
isLoggedIn: false,
}
},
created() {
if (window.Laravel.isLoggedin) {
this.isLoggedIn = true
}
},
methods: {
logout(e) {
console.log('ss')
e.preventDefault()
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.post('/api/logout')
.then(response => {
if (response.data.success) {
window.location.href = "/"
} else {
console.log(response)
}
})
.catch(function (error) {
console.error(error);
});
})
}
},
}
</script>
Create Vue Pages
Navigate to resources>js folder and create a folder called pages. In side pages folder, let’s create these pages:
Homepage:
<template>
<div>
Homepage
</div>
</template>
<script>
export default {
name: "Home",
data() {
return {
//
}
},
created() {
},
methods: {}
}
</script>
Regitration page:
<template>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="alert alert-danger" role="alert" v-if="error !== null">
{{ error }}
</div>
<div class="card card-default">
<div class="card-header">Register</div>
<div class="card-body">
<form>
<div class="form-group row">
<label for="name" class="col-sm-4 col-form-label text-md-right">Name</label>
<div class="col-md-6">
<input id="name" type="email" class="form-control" v-model="name" required
autofocus autocomplete="off">
</div>
</div>
<div class="form-group row">
<label for="email" class="col-sm-4 col-form-label text-md-right">E-Mail Address</label>
<div class="col-md-6">
<input id="email" type="email" class="form-control" v-model="email" required
autofocus autocomplete="off">
</div>
</div>
<div class="form-group row">
<label for="password" class="col-md-4 col-form-label text-md-right">Password</label>
<div class="col-md-6">
<input id="password" type="password" class="form-control" v-model="password"
required autocomplete="off">
</div>
</div>
<div class="form-group row mb-0">
<div class="col-md-8 offset-md-4">
<button type="submit" class="btn btn-primary" @click="handleSubmit">
Register
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
name: "",
email: "",
password: "",
error: null
}
},
methods: {
handleSubmit(e) {
e.preventDefault()
if (this.password.length > 0) {
axios.get('/sanctum/csrf-cookie').then(response => {
axios.post('api/register', {
name: this.name,
email: this.email,
password: this.password
})
.then(response => {
if (response.data.success) {
window.location.href = "/login"
} else {
this.error = response.data.message
}
})
.catch(function (error) {
console.error(error);
});
})
}
}
},
beforeRouteEnter(to, from, next) {
if (window.Laravel.isLoggedin) {
return next('dashboard');
}
next();
}
}
</script>
Login page:
<template>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="alert alert-danger" role="alert" v-if="error !== null">
{{ error }}
</div>
<div class="card card-default">
<div class="card-header">Login</div>
<div class="card-body">
<form>
<div class="form-group row">
<label for="email" class="col-sm-4 col-form-label text-md-right">E-Mail Address</label>
<div class="col-md-6">
<input id="email" type="email" class="form-control" v-model="email" required
autofocus autocomplete="off">
</div>
</div>
<div class="form-group row">
<label for="password" class="col-md-4 col-form-label text-md-right">Password</label>
<div class="col-md-6">
<input id="password" type="password" class="form-control" v-model="password"
required autocomplete="off">
</div>
</div>
<div class="form-group row mb-0">
<div class="col-md-8 offset-md-4">
<button type="submit" class="btn btn-primary" @click="handleSubmit">
Login
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
email: "",
password: "",
error: null
}
},
methods: {
handleSubmit(e) {
e.preventDefault()
if (this.password.length > 0) {
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.post('api/login', {
email: this.email,
password: this.password
})
.then(response => {
console.log(response.data)
if (response.data.success) {
this.$router.go('/dashboard')
} else {
this.error = response.data.message
}
})
.catch(function (error) {
console.error(error);
});
})
}
}
},
beforeRouteEnter(to, from, next) {
if (window.Laravel.isLoggedin) {
return next('dashboard');
}
next();
}
}
</script>
Dashboard:
<template>
<div>
Welcome {{ name }}
</div>
</template>
<script>
export default {
name: "Dashboard",
data() {
return {
name: null,
}
},
created() {
if (window.Laravel.user) {
this.name = window.Laravel.user.name
}
},
beforeRouteEnter(to, from, next) {
if (!window.Laravel.isLoggedin) {
window.location.href = "/";
}
next();
}
}
</script>
About page:
<template>
<div>
About us
</div>
</template>
<script>
export default {
name: "About"
}
</script>
Create Vue Components
Go to resources>js>components folder and create 3 Vue components.
Books component:
<template>
<div>
<h4 class="text-center">All Books</h4><br/>
<table class="table table-bordered">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Author</th>
<th>Created At</th>
<th>Updated At</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="book in books" :key="book.id">
<td>{{ book.id }}</td>
<td>{{ book.name }}</td>
<td>{{ book.author }}</td>
<td>{{ book.created_at }}</td>
<td>{{ book.updated_at }}</td>
<td>
<div class="btn-group" role="group">
<router-link :to="{name: 'editbook', params: { id: book.id }}" class="btn btn-primary">Edit
</router-link>
<button class="btn btn-danger" @click="deleteBook(book.id)">Delete</button>
</div>
</td>
</tr>
</tbody>
</table>
<button type="button" class="btn btn-info" @click="this.$router.push('/books/add')">Add Book</button>
</div>
</template>
<script>
export default {
data() {
return {
books: []
}
},
created() {
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.get('/api/books')
.then(response => {
this.books = response.data;
})
.catch(function (error) {
console.error(error);
});
})
},
methods: {
deleteBook(id) {
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.delete(`/api/books/delete/${id}`)
.then(response => {
let i = this.books.map(item => item.id).indexOf(id); // find index of your object
this.books.splice(i, 1)
})
.catch(function (error) {
console.error(error);
});
})
}
},
beforeRouteEnter(to, from, next) {
if (!window.Laravel.isLoggedin) {
window.location.href = "/";
}
next();
}
}
</script>
Add book component:
<template>
<div>
<h4 class="text-center">Add Book</h4>
<div class="row">
<div class="col-md-6">
<form @submit.prevent="addBook">
<div class="form-group">
<label>Name</label>
<input type="text" class="form-control" v-model="book.name">
</div>
<div class="form-group">
<label>Author</label>
<input type="text" class="form-control" v-model="book.author">
</div>
<button type="submit" class="btn btn-primary">Add Book</button>
</form>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
book: {}
}
},
methods: {
addBook() {
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.post('/api/books/add', this.book)
.then(response => {
this.$router.push({name: 'books'})
})
.catch(function (error) {
console.error(error);
});
})
}
},
beforeRouteEnter(to, from, next) {
if (!window.Laravel.isLoggedin) {
window.location.href = "/";
}
next();
}
}
</script>
Edit book component:
<template>
<div>
<h4 class="text-center">Edit Book</h4>
<div class="row">
<div class="col-md-6">
<form @submit.prevent="updateBook">
<div class="form-group">
<label>Name</label>
<input type="text" class="form-control" v-model="book.name">
</div>
<div class="form-group">
<label>Author</label>
<input type="text" class="form-control" v-model="book.author">
</div>
<button type="submit" class="btn btn-primary">Update Book</button>
</form>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
book: {}
}
},
created() {
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.get(`/api/books/edit/${this.$route.params.id}`)
.then(response => {
this.book = response.data;
})
.catch(function (error) {
console.error(error);
});
})
},
methods: {
updateBook() {
this.$axios.get('/sanctum/csrf-cookie').then(response => {
this.$axios.post(`/api/books/update/${this.$route.params.id}`, this.book)
.then(response => {
this.$router.push({name: 'books'});
})
.catch(function (error) {
console.error(error);
});
})
}
},
beforeRouteEnter(to, from, next) {
if (!window.Laravel.isLoggedin) {
window.location.href = "/";
}
next();
}
}
</script>
In the three files, we have used Axios to call Laravel API & check auth using sanctum.
Define Vue Routes
In the resources>js folder, create a folder called router. Inside router directory, make a file named index.js and paste this code:
import {createWebHistory, createRouter} from "vue-router";
import Home from '../pages/Home';
import About from '../pages/About';
import Register from '../pages/Register';
import Login from '../pages/Login';
import Dashboard from '../pages/Dashboard';
import Books from '../components/Books';
import AddBook from '../components/AddBook';
import EditBook from '../components/EditBook';
export const routes = [
{
name: 'home',
path: '/',
component: Home
},
{
name: 'about',
path: '/about',
component: About
},
{
name: 'register',
path: '/register',
component: Register
},
{
name: 'login',
path: '/login',
component: Login
},
{
name: 'dashboard',
path: '/dashboard',
component: Dashboard
},
{
name: 'books',
path: '/books',
component: Books
},
{
name: 'addbook',
path: '/books/add',
component: AddBook
},
{
name: 'editbook',
path: '/books/edit/:id',
component: EditBook
},
];
const router = createRouter({
history: createWebHistory(),
routes: routes,
});
export default router;
Import All to app.js
We are about to finish. This is the last step. Open/create app.js from resources>js folder and paste this code:
import {createApp} from 'vue'
require('./bootstrap')
import App from './App.vue'
import axios from 'axios'
import router from './router'
const app = createApp(App)
app.config.globalProperties.$axios = axios;
app.use(router)
app.mount('#app')
In this file, we have imported all necessary dependencies, routes etc.
The Output
We have completed all the tasks. Let’s run the project and see the output. I always open 3 terminals. One for Laravel, one for Vue and another for running commands.
# terminal 1
php artisan serve
# terminal 2
npm run watch
# terminal 3
run all commands
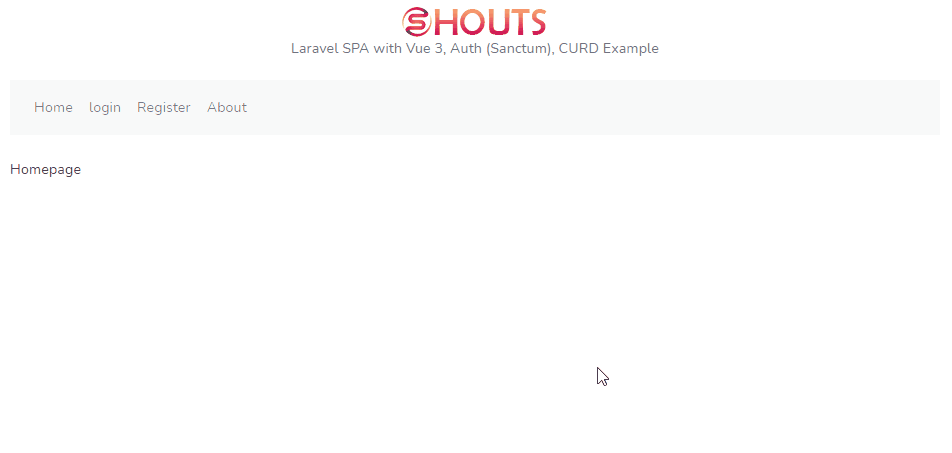
That’s all. You can download this project from GitHub. Thanks for reading. 🙂
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.