Laravel Login Event Listener
Sometimes we want to display a welcome back message, want to store log data etc. on successful login. In Laravel, we can do this very easily. Let’s follow these steps:
Last tested on Laravel 6.2
Table of Contents
Step 1 : Create a Listener
We need to create a listener. To make a listener run this artisan command:
php artisan make:listener LoginSuccessful --event=Illuminate\Auth\Events\Login
This will specify the name of the login event on creating the listener. We can also create a listener without specifying any event.
Navigate to the app>Listeners
directory and open LoginSuccessful.php file. We are going to define a message in the handle()
method. We need to use use Session;
for this.
So the full LoginSuccessful.php looks like:
<?php
namespace App\Listeners;
use Illuminate\Auth\Events\Login;
use Session;
class LoginSuccessful
{
/**
* Create the event listener.
*
* @return void
*/
public function __construct()
{
//
}
/**
* Handle the event.
*
* @param Login $event
* @return void
*/
public function handle(Login $event)
{
Session::flash('login-success', 'Hello ' . $event->user->name . ', welcome back!');
}
}
Step 2 : Map the Listener
Now we have to attach this listener to EventServiceProvider. Open app>Providers>EventServiceProvider.php
file and add this line to $listen
property:
protected $listen = [
// ------------- ,
'Illuminate\Auth\Events\Login' => ['App\Listeners\LoginSuccessful'],
];
Step 3 : Display Message
We have completed all the necessary things. It’s time to display the message in blade template. So open your welcome blade template. My welcome template is home.blade.php
. I’ve opened it from resources>views
folder.
We have add this in the welcome template:
@if(session('login-success'))
<div class="alert alert-success" role="alert">
{{ session('login-success') }}
</div>
@endif
So, my full home.blade.php looks like:
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">Dashboard</div>
<div class="card-body">
@if(session('login-success'))
<div class="alert alert-success" role="alert">
{{ session('login-success') }}
</div>
@endif
@if (session('status'))
<div class="alert alert-success" role="alert">
{{ session('status') }}
</div>
@endif
You are logged in!
</div>
</div>
</div>
</div>
</div>
@endsection
Let’s run this project and see the output. After successfully logged-in, I’ve shown this welcome message in my welcome view:
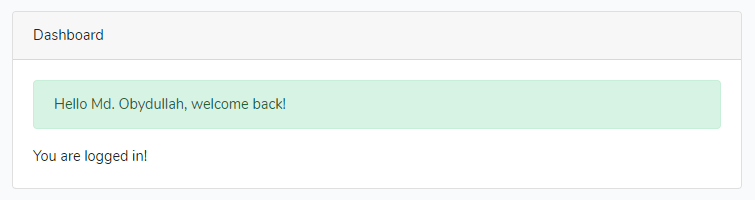
We are done. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.